How do I grab user input with my Discord bot in Python?
If you want the bot to just send the message of the user without the command part ("!id") you'll have to specify which part of the string you want to send back. Since strings are really just lists of characters you can specify what part of the string you want by asking for an index.
For example:
"This is an example"[1]
returns "h".
In your case you just want to get rid of the beginning part of the string which can be done like so:
@client.event
async def on_message(message):
if message.content.startswith("!id"):
await client.send_message(message.channel, message.content[3:])
this works because it sends the string back starting with the 4th index place (indexes start at 0 so really [3] is starting at the fourth value)
This also applies if you just want to save the user's message as a variable as you can just do this:
userInput = message.content[3:]
You read more about string manipulation at the python docs.
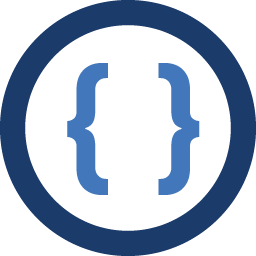
Admin
Updated on June 28, 2022Comments
-
Admin almost 2 years
I would like to grab user input from the user when they enter a certain command and store the input into a variable (but not the command) so that I can do things with it.
As an example, when the user types "!id 500", I want the bot to type "500" in the channel. I have tried just using message.content but it doesn't work properly because the bot spams it indefinitely, plus it shows the command as well.
@client.event async def on_message(message): if message.content.startswith("!id"): await client.send_message(message.channel, message.content)
Sorry if this is a silly question, but any help would mean a lot.