How to set channel object using the channel id in discord.py?
18,008
Solution 1
You can get the channel object either by ID or by name.
Use discord.utils.get()
to return a channel by its name (example for voice channels):
channel = discord.utils.get(server.channels, name="Channel_name_here", type="ChannelType.voice")
Or you can use discord.get_channel(id)
if you have the ID of the channel.
So for example if you have a list of channel names you want to check:
channel_names = ['channel1', 'channel2', 'channel3']
for ch in channel_names:
channel = discord.utils.get(server.channels, name=ch, type="ChannelType.voice")
full(channel)
See more in the documentation:
Solution 2
If you want this to be a command, you can also take advantage of converters:
@bot.command(pass_context=True)
async def full(ctx, channel: discord.Channel):
if len(channel.voice_members) == channel.user_limit:
await bot.say("{} is full".format(channel.name))
else:
await bot.say("{} is not full".format(channel.name))
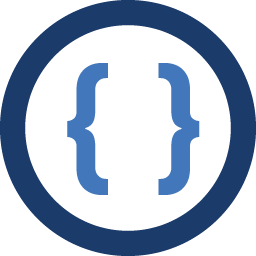
Author by
Admin
Updated on June 07, 2022Comments
-
Admin almost 2 years
Hello guys I'm trying to make a function that checks if few channels in the my discord server are full but I didn't know how to specify them. The best idea I had was using the idea but couldn't set it to a channel object so how can I do that or is there any other good ideas?
def full(channel): while True: if len(channel.voice_members) == channel.user_limit: print("Wooo There is a full channel.") asyncio.sleep(10)
But I didn't know what to set the channel parameter. Thanks in advance for your help.