How do i keep the header cell moving with the tableview cells in Swift 2.0
Solution 1
GO TO STORYBOARD > SELECT VIEW > SELECT TABLEVIEW > PROPERTY INSPECTOR > CHNAGE STYLE TO GROUPPED from PLAIN
Don't forget to handle footerview. In Groupped mode a footer will appear and arrange background or other properties. They will change.
Solution 2
You can do it using sections. If i understood your question correctly, what you want to do is, you have to display header in the middle of screen while having some cells above the header and some cells below the header, and when you scroll the tableView up your header should be scrolled to top and when it reaches to the top you want your header to keep at the top while cells should be scrolled underneath the header.
So to do this return two section from the data source
func numberOfSectionsInTableView(tableView: UITableView) -> Int
{
return 2
}
And in the data source return conditional cells
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int
{
If(section == 1) {
return <Return number of cell you want to display above header>
} else {
return <Return number of cell you want to display below header>
}
}
Then override the delegate
func tableView(tableView: UITableView, viewForHeaderInSection section: Int) -> UIView?
{
if(section == 0) {
return nil //As you do not have to display header in 0th section, return nil
} else {
return <Return your header view>
//You can design your header view inside tableView as you design cell normally, set identifier to it and dequeue cell/headerView as:
//However this may result in unexpected behavior since this is not how the tableview expects you to use the cells.
let reuseIdentifier : String!
reuseIdentifier = String(format: "HeaderCell")
let headerView = tableView.dequeueReusableCellWithIdentifier(reuseIdentifier, forIndexPath: NSIndexPath(forRow: 0, inSection: 0))
return headerView
}
}
Set conditional height as
func tableView(tableView: UITableView, heightForHeaderInSection section: Int) -> CGFloat
{
if(section == 0) {
return 0
} else {
return <Return your header view height>
}
}
What we are doing here is, we are displaying header in second section which would have some cell, so you will see some cells in the tableView without header and below this cells you will see headerView with some cells and when you scroll your tableView up headerView will scroll with the cell till it reaches at the top.
Hope this will help you.
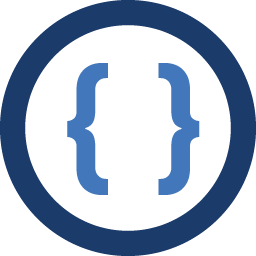
Admin
Updated on August 29, 2020Comments
-
Admin over 3 years
I am trying to create a tableview which starts the header in the middle of the tableview and then can be scrolled up with the added tableView Cells to the top then stops, and then the tableview cells can just scroll "under" it. I got the header to be in the middle of the tableview, but the tableView Cells just scroll on top of it, and the header doesnt really scroll to the top. it just stay where it is. I want to be able to let the header move as you scroll the list, and then stops when it reaches the top of the list, and then its just the tableView Cells that are moving afterwards.
I am using the latest version of XCode
-
Devang Tandel over 6 yearsPlease feel free to comment for down votes, i will appreciate
-
Devang Tandel over 6 yearslet me how it is not helping you, i might be able to help you with it
-
SafeFastExpressive over 6 yearsI didn't down-vote you, but this is an example of a poor answer. First, your instructions are in all caps and have multiple misspellings. But much more importantly, your instructions don't describe why the reader expect them to work. What is it about grouped style that's going to change header behavior?
-
Devang Tandel over 6 years@RandyHill - my answer does work. See it worked for 9 people. Still thank you for the comment
-
Mamta about 6 years@Dev_Tandel This solves the header scrolling problem. But when we change style to grouped, the distance between sections increase. How to make it look like the plain style?
-
Devang Tandel about 6 years@Mamta - Try changing the height of footer and header. By default it will be 18
-
Devang Tandel about 6 years@Mamta - try programmatically once if not get result through inspector
-
Mo Zaatar about 6 yearsActually this one saved my life. I have a tableview with 11 instance of a custom cell of my design. They all have headers/footers. Scrolling and editing the cells was behaving wrong (some of the issues I have were focusing on header not the editable text field and closing the keyboard doesn't trigger the re-render) Changing the style from Plain to Grouped fixed all of these issues
-
fujianjin6471 almost 5 yearsAfter 5 years of iOS development, I finally know the difference between plain and group, tks!