How do I pass data between pages in PHP?
Solution 1
PHP is stateless unless you save things in session (which isn't secure right?)
You would need to make page2.php read all the values from page1.php and store them either in a server side state (session) or a client state (cookies or maybe hidden fields in the form)
If you want any of this secure or a secret, then you have to consider all of that as well. Nothing I explained is secret or secure in any way.
EDIT: here is an example of page1.php that sends the values of page1.php to page2.php as get parameters. You can do this with hidden fields, cookies or sessions as well.
What is important to remember is that page2.php is totally unaware of page1.php, and can't get to the values like you could it forms programming. Each page starts and ends it's life by the time you see a full web page, so you have to use some extra tricks to keep values. Those tricks are sessions, cookies, form posts, or gets.
<html>
<head>
<title>Page 1</title>
</head>
<body>
<?php
//set defaults assuming the worst
$total = 0;
$one =0;
$two=0;
//verify we have that value in $__POST
if (isset($_POST['submit']))
{
$submit = $_POST['submit'];
//If it is true, try some math
if($submit == "sub-total")
{
if (isset($_POST['one']))
{
$one = $_POST['one'];
//Add checks to see if your values are numbers
if ( ! is_numeric($one)) { $one = 0;}
}
if (isset($_POST['two']))
{
$two = $_POST['two'];
if ( ! is_numeric($two)) { $two = 0;}
}
$total = $one + $two;
echo " Your Price is \$ " .number_format ($total, 2, '.', ','). "<BR>";
}
if($submit == "submit" )
{
//go to page two, with the total from page1.php passed as a $__GET value
header("Location: page2.php?total=".$total);
}
}
?>
<form method="post" action="page1.php?submit=true">
<input type="text" name="one" id="one" value="<?=$one?>"/>
<input type="text" name="two" id="two" value="<?=$two?>"/>
<input type="submit" name="submit" value="sub-total" />
<input type="submit" name="submit" value="submit" />
</form>
</body>
</html>
Solution 2
You can try using $_REQUEST
instead of $_GET
or $_POST
- it works for both. Also, if you put <input type='hidden'
in the form on page 2 with your variables posted from page 1 they should get passed right along.
Solution 3
your php pages don't have state, so you have to get the posted variables, and send them along via hidden input fields.
<input type="hidden".....
You could also use sessions ,but it is a bit hackish to use them with forms if you ask me.
Solution 4
It depends on the method
attribute of your HTML form
how the variables get passed to your PHP script.
In this case, it sounds like page1.php
does not have any side effect, and just passes on values, so the form on page1.php
should use method=get
, and the code of page2.php
that reads these fields can find them in $_GET
.
The Submit button on page2.php
(presumably) does have side effects on the server side, so it should use method=post
, which you can read back from $_POST
on the server side.
Also, have a look at the HTML source of page2.php
after filling out the first page, to see if the values are passed correctly.
Solution 5
little late on the answer, yes, best way is using type=hidden
as others have mentioned already.
page1 sends _POST to page2, which "saves" all of it in hidden fields, so it can send everything together to the final (or another) page.
but more importantly, and going to a broader-topic, is considering why using pages at all to divide a form!
sorry for touching this subject here, I just felt like giving my 2 cents.
don't page forms
I'd say best thing is avoid paging as much as possible and only good reasons to do it so would be if you need server validation and backwards compatibility with browsers that won't accept javascript.
use newer resources instead
layout and design can both be achieved using javascript and CSS, which are both meant for that. even if you do the user to "press next", use dhtml and all in 1 file. need server validation? ajax.
unless you're using HTML frames (which you probably shouldn't), loading a whole page is a redundant and unnecessary server and client load that takes more time in both using and programming (to take care of passing form info from one place to another).
and it's far from being the best way to save the state of what's being done. granted, this is a whole complicated subject on its own, but again, ajax would be best here.
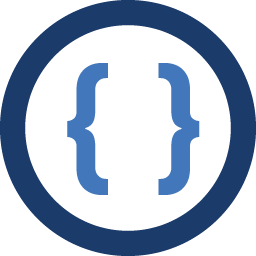
Admin
Updated on August 06, 2020Comments
-
Admin over 3 years
In a nutshell on "page1.php" I have a calculator that consists of an HTML form, and then the PHP code totals the input and displays the total price. Below the price, it also displays a link to "page2.php" which contains an HTML form where they can enter their contact information. Upon submitting the form the selections they made on "page1.php" in the pricing calculator as well as the contact info on "page2.php" are emailed to me, and they are redirected to the home page.
In the email that is submitted to me, I receive the contact info from "page2.php", but I don't receive anything from "page1.php", so the variables are not getting correctly passed. In addition to the PHP on each page, I am using hidden values in an HTML form on "page2.php" to echo the data that was entered in the HTML form on "page1.php". I know that one of my issues is that I have a couple of
$_GET
fields when my form is "post".However when I change it so that everything is
$_POST
, the calculator no longer works. I tried to put this altogether with different snippets of code suggested by others. The form on "page1.php" has 13 fields, named "one" - "thirteen". $total display the values of 1-13.<?php $submit = $_GET['submit']; if($submit == "true") { $total = ($_POST['one'] + $_POST['two'] + $_POST['three'] + $_POST['four'] + $_POST['five'] + $_POST['six'] + $_POST['seven'] + $_POST['eight']+ $_POST['nine'] + $_POST['ten']+ $_POST['eleven'] + $_POST['twelve']+ $_POST['thirteen']); echo " Your Price is \$ " .number_format ($total, 2, '.', ','). "<BR>"; echo ('">Get Your Project Started</a>'); } ?>
The second form uses hidden values to echo the info from page1.php, and has three more fields named "name", "email" and "details".
<?php $to = "[email protected]"; $message = "Pages:\t$_POST[one]\n"; $message .= "Pages:\t$_POST[two]\n"; $message .= "Pages:\t$_POST[three]\n"; $message .= "Ecommerce:\t$_POST[four]\n"; $message .= "No Ecommerce:\t$_POST[five]\n"; $message .= "CMS:\t$_POST[six]\n"; $message .= "No CMS:\t$_POST[seven]\n"; $message .= "Audio or Video:\t$_POST[eight]\n"; $message .= "Flash Intro:\t$_POST[nine]\n"; $message .= "Image Gallery:\t$_POST[ten]\n"; $message .= "Graphic Design or Logo:\t$_POST[eleven]\n"; $message .= "Copy:\t$_POST[twelve]\n"; $message .= "Images:\t$_POST[thirteen]\n"; $message .= "Price Total:\t$_POST[total]\n"; $message .= "Name:\t$_POST[name]\n"; $message .= "Email:\t$_POST[email]\n"; $message .= "\n"; $message .= "\n"; $message .= "Details:\t$_POST[details]\n"; mail($to, $subject, $message, $headers) ; } ?>
So what would be the correct PHP to put on "page1.php" and "page2.php"? Sorry the code is such a mess, if anyone could point me in the right direction, that would be great.