Storing Form Data as a Session Variable
Solution 1
To use session variables, it's necessary to start the session by using the session_start
function, this will allow you to store your data in the global variable $_SESSION
in a productive way.
so your code will finally look like this :
<strong>Test Form</strong>
<form action="" method"post">
<input type="text" name="picturenum"/>
<input type="submit" name="Submit" value="Submit!" />
</form>
<?php
// starting the session
session_start();
if (isset($_POST['Submit'])) {
$_SESSION['picturenum'] = $_POST['picturenum'];
}
?>
<strong><?php echo $_SESSION['picturenum'];?></strong>
to make it easy to use and to avoid forgetting it again, you can create a session_file.php
which you will want to be included in all your codes and will start the session for you:
session_start.php
<?php
session_start();
?>
and then include it wherever you like :
<strong>Test Form</strong>
<form action="" method"post">
<input type="text" name="picturenum"/>
<input type="submit" name="Submit" value="Submit!" />
</form>
<?php
// including the session file
require_once("session_start.php");
if (isset($_POST['Submit'])) {
$_SESSION['picturenum'] = $_POST['picturenum'];
}
?>
that way it is more portable and easy to maintain in the future.
other remarks
if you are using Apache version 2 or newer, be careful. instead of
<?
to open php's tags, use<?php
, otherwise your code will not be interpretedvariables names in php are case-sensitive, instead of write $_session, write $_SESSION in capital letters
good work!
Solution 2
That's perfectly fine and will work. But to use sessions you have to put session_start();
on the first line of the php code. So basically
<?php
session_start();
//rest of stuff
?>
Solution 3
Yes this is possible. kizzie is correct with the session_start();
having to go first.
another observation I made is that you need to filter your form data using:
strip_tags($value);
and/or
stripslashes($value);
Solution 4
You can solve this problem using this code:
if(!empty($_GET['variable from which you get']))
{
$_SESSION['something']= $_GET['variable from which you get'];
}
So you get the variable from a GET form, you will store in the $_SESSION['whatever']
variable just once when $_GET['variable from which you get']
is set and if it is empty $_SESSION['something']
will store the old parameter
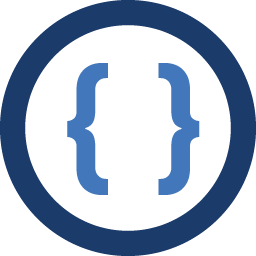
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
So I was wondering if it would be possible to store data coming in from a form as a session variable.
Heres what I have so far, but I don't know what to put for the Form Action.
Thanks for looking!
<strong>Test Form</strong> <form action="" method"post"> <input type="text" name="picturenum"/> <input type="submit" name="Submit" value="Submit!" /> </form> <? if (isset($_POST['Submit'])) { $_session['picturenum'] = $_POST['picturenum']; } ?> <strong><? echo $_session['picturenum'];?></strong>
-
Admin over 13 yearsSo basically this just sanitizes the data?
-
NullUserException over 13 years-1 This is a totally useless answer.
stripslashes()
is useless now that magic quotes are off by default (and also this would "de-sanitize" your data if anything). Also why would you want to dostrip_tags()
(orstripslashes()
) on information you save to sessions? -
Todd Moses over 13 yearsYou cannot be guaranteed that magic quotes are off. I have worked with too many clients who used hosting they had no control over and magic quotes was on. It is a best practice to assume nothing. What if the code is moved or the host changes the settings, etc...
-
Todd Moses over 13 yearsIf the data is coming from the user then you want to treat it as dangerous. Where is that data going to go - maybe the DB, etc... The point is to protect your site. Because down the road another developer may be working on the site and use the user data for something else without checking for filtering.
-
Andy Lobel over 10 yearshtmlspecialchars for displaying data, prepare statements for inserting into db ;/
-
Amyunimus over 10 yearsIs it possible to retrieve input values using their id rather than name?
-
Michael Kent over 9 years# in action will reload the current page which is what you want it to do so the php runs.
-
BeNice over 8 yearsDumb question. If you start a session and then do (accidentally) another
session_start();
does that wipe all the data of the first session? -
Fopa Léon Constantin over 8 years@OldMauiMan, call
session_start();
(accidentally) twice in the same code will not do anything wrong. The only way to wipe out all the data is to usesession_destroy();
-
BeNice over 8 yearsThanks for that. I know people don't like that but how do you say thank you for helpful info here? (I am British and CANNOT not say thank you).
-
Fopa Léon Constantin over 8 years@OldMauiMan, Profitable way is to vote you their answer (the number in the left of the answer title). However, just says thank is fine for me ;-)
-
Fopa Léon Constantin over 8 years@Amyunimus, It is not possible to retrieve value using their id, because those id are not send in the
$_POST
variable in the server side. They are only usefull in the client side (using javascript). -
mario64 almost 8 yearsWhen I changed the button from an input element to a button element, the value returned by $_SESSION is then used as the value of <button>. Any idea why?