How do properties work in Object Oriented MATLAB?
Solution 1
Using a Vanilla Class
When using vanilla class you need to tell Matlab to store a modified copy of the object to save the changes in the property value. So,
>> a=testprop
>> a.Request(5); % will NOT change the value of a.numRequests.
5
>> a.Request(5)
5
>> a.numRequests
ans =
0
>> a=a.Request; % However, this will work but as you it makes a copy of variable, a.
5
>> a=a.Request;
5
>> a.numRequests
ans =
2
Using the Handle Class
If you inherit from the handle class, that is
classdef testprop < handle
then you can write,
>> a.Request(5);
>> a.Request(5);
>> a.numRequests
ans =
2
Update: Using Vanilla Class
As Kamran notes for the above to work the definition of the Request
method in the question's example code needs to be changed to include an output argument of type testprop.
Thanks Kamran.
Solution 2
You have to remember that syntactically in Matlab, you're probably closer to C, than C++ or Java, at least with respect to objects. So, of you want to change the "contents" of a value object (really just a special struct
), you need to return the object from the function.
Azim was correct to point out that if you want Singleton behavior (which, from your code, you seem to), you need to use a "handle" class. Instances of classes that derive from Handle all point to a single instance, and operate only on it.
You can read more about the differences between Value and Handle classes.
Solution 3
I made the class testprop and tried to excute the code which Azim suggested but it did not work. When I executed the following command:
a=a.Request(1)
The following error was generated:
??? Error using ==> Request Too many output arguments.
I think the problem is that we did not determine any output when declaring Request method. So we should change it to:
function this = Request(this, val)
and now:
>> a = testprop;
>> a = a.Request(1);
>> a.numRequests
ans = 1
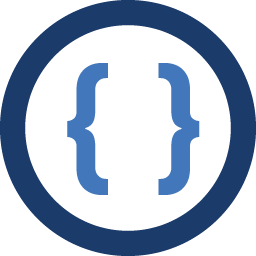
Admin
Updated on June 16, 2022Comments
-
Admin almost 2 years
I am trying to create a MATLAB class with a member variable that's being updated as a result of a method invocation, but when I try to change the property within the class it (apperently, from what I understood from MATLAB's memory management) creates a copy of the object and then modifies it, leaving the original object's property untouched.
classdef testprop properties numRequests=0; end methods function Request(this, val) disp(val); this.numRequests=this.numRequests+1; end end end
.
>> a=testprop; >> a.Request(9); >> a.Request(5); >> a.numRequests ans = 0