How do you debug CodeIgniter applications?
Solution 1
You can use Xdebug and it's a good idea to start with Test Driven Development, in PHP you can use PHP Unit for that.
Solution 2
A good practice is to enclose critical code into try/catch blocks so that you can handle exceptions, and then log the stack trace of that error. In my recent experience, I've used try/catch blocks along with show_error() function provided by CodeIgniter to debug and catch errors in my code. Here's an example:
public function getData()
{
//Query the data table for every record and row
try{
$query = $this->db->get('accounts');
if ($query->num_rows() > 0)
{
show_error('Database is empty!');
}
else
{
return $query->result();
}
} catch(Exception $e){
log_message('debug',$e->getMessage()); // use codeigniters built in logging library
show_error($e->getMessage()); // or echo $e->getMessage()
}
}
PHP Exception class provides various methods to help debug your error. http://www.php.net/manual/en/class.exception.php
Solution 3
If you've not already looked at the amazing web development extension for firefox called Firebug you should check it out!
Firebug has an extension called FirePHP which enables you to debug PHP applications. Someone has also made an plugin fore CodeIgniter called FireIgnition.
It enables you log variables and so forth making it easier to see what is going on as pages are being executed.
Solution 4
I find dbug to be quite helpful when I need to throw a variable, object, array, or resource on the screen to view it:
An article for integrating it into CodeIgniter can be found here.
Solution 5
You did if ($query->num_rows() > 0)
. This will print an error if there are MORE THAN 0 rows returned.
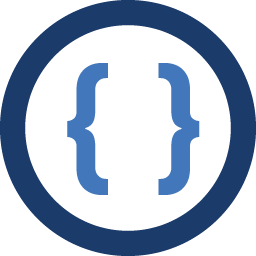
Admin
Updated on July 24, 2022Comments
-
Admin almost 2 years
I followed steps in a tutorial to learn how to use Code Igniter to build a PHP/MySQL application and upon completion the application fails with no error. I'm using TextMate rather than a full featured IDE.
How do most developers using Code Igniter debug their applications? Is there something in the framework for bubbling errors and a stack trace into the browser session?
The specific problem I'd like to address now seems database related. I configured my database, setup autoload.php, and created a controller and model for handling table data, and a view for presenting it. From my accounts_model.php:
public function getData() { //Query the data table for every record and row $query = $this->db->get('accounts'); if ($query->num_rows() > 0) { show_error('Database is empty!'); } else { return $query->result(); } }
When I run the application, I see "An Error was Encountered\nDatabase is empty!". Since I know the database is not empty and configuration is correct, I need something from the framework to give me a hint whether it is connecting to the database or why the query is empty.
-
Admin over 13 yearsWell, radda radda! Thank you! I copied that code from the tutorial and overlooked that fine detail. That gets me past one error and into another. I'm just guessing here that most folks quickly graduate to using a full IDE that allows viewing stack trace, stepping through code, etc.
-
gen_Eric over 13 yearsI don't use an IDE that allows any of those things (well, not for PHP anyway). :-P
-
Admin over 13 yearsWorked through the next error to, but thanks for asking. With my experience level so far, I can't imagine developing a large application using just TextMate. I'm familiar with FlexUnit and thus have some experience unit testing; hence, I selected Enrico Pallazzo's suggestions. Years ago I setup Eclipse and PDT and tested Xdebug. It was all cumbersome compared to tools like Visual Studio, which I was familiar with at the time. I'm hoping to come across some good practices and tools as I dive into more PHP development.
-
Wouter Dorgelo almost 12 yearsUpdate (2 years later): PHP Unit works pretty well with Selenium seleniumhq.org (functional testing)
-
bg17aw over 8 yearsSeems like the link is dead: http://www.lastcraft.com/first_test_tutorial.php