How do you Make A Repeat-Until Loop in C++?
Solution 1
do
{
// whatever
} while ( !condition );
Solution 2
When you want to check the condition at the beginning of the loop, simply negate the condition on a standard while
loop:
while(!cond) { ... }
If you need it at the end, use a do
... while
loop and negate the condition:
do { ... } while(!cond);
Solution 3
You could use macros to simulate the repeat-until syntax.
#define repeat do
#define until(exp) while(!(exp))
Solution 4
For an example if you want to have a loop that stopped when it has counted all of the people in a group. We will consider the value X to be equal to the number of the people in the group, and the counter will be used to count all of the people in the group. To write the
while(!condition)
the code will be:
int x = people;
int counter = 0;
while(x != counter)
{
counter++;
}
return 0;
Solution 5
Just use:
do
{
//enter code here
} while ( !condition );
So what this does is, it moves your 'check for condition' part to the end, since the while
is at the end. So it only checks the condition after running the code, just like how you want it
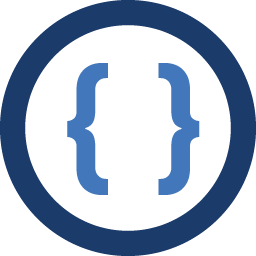
Admin
Updated on March 01, 2021Comments
-
Admin about 3 years
How do you Make A Repeat-Until Loop in C++? As opposed to a standard While or For loop. I need to check the condition at the end of each iteration, rather than at the beginning.