How to find sum of several integers input by user using do/while, While statement or For statement
105,317
The FOR loop worked well, I modified it a tiny bit:
#include<iostream>
using namespace std;
int main ()
{
int sum = 0;
int number;
int numberitems;
cout << "Enter number of items: \n";
cin >> numberitems;
for(int i=0;i<numberitems;i++)
{
cout << "Enter number: \n";
cin >> number;
sum=sum+number;
}
cout<<"sum is: "<< sum<<endl;
}
HOWEVER, the WHILE loop has got some errors on line 11 (Count was not declared in this scope). What could be the issue? Also, if you would have a solution using DO,WHILE loop it would be wonderful. Thanks
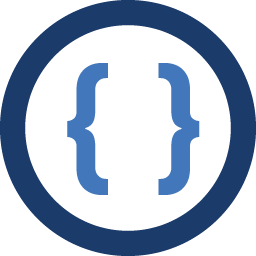
Author by
Admin
Updated on November 06, 2020Comments
-
Admin over 3 years
Please help out here. I want to create a program whereby a user inputs several numbers (let's say 6 numbers from his/ her head). The program should then go ahead and calculate the sum of all these numbers. I however have to use a loop statement, either For statement, While statement or do/while statement. This is what I have so far:
#include <iostream> using namespace std; int main() { int count = 1; int sum = 0; int number; int numberitems; cout << "Enter number of items: \n"; cin >> numberitems; cout << "Enter number: \n"; cin >> number; do { sum = sum + number; count++; } while (count <= 6); }
AND HERE IS THE OTHER CODE USING WHILE STATEMENT
#include <iostream> using namespace std; int main() { int count = 1; int sum = 0; int number; cout << "Enter number: \n"; cin >> number; while (count <= 6) { sum = sum += number; count++; } cout << sum << endl; }
I know this is beginner stuff, well am a beginner so help out a sister politely. THANKS
-
John St about 10 yearsWith the first way (the best) you don't need a while loop or the variable count. At your code what you did is that you gave the number once. Now, with a loop, you give as many numbers as "numberitems" is. If this helped you please up-vote and accept my answer (i need some reputation to comment on other peoples posts, and help them with their problems) Thanks! .
-
Nathan Tuggy about 9 yearsPlease use the Post answer button only for actual answers. You should modify your original question to add additional information, or perhaps ask another to avoid making this a chameleon question.
-
Nathan Tuggy about 9 yearsGenerally, answers are much more helpful if they include an explanation of what the code is intended to do, and why that solves the problem without introducing others. (This post was flagged by at least one user, presumably because they thought an answer without explanation should be deleted.)