How get moving text in widget with given width
6,696
Solution 1
You can do something like this:
import 'dart:async';
import 'package:flutter/material.dart';
class ScrollingText extends StatefulWidget {
final String text;
final TextStyle textStyle;
final Axis scrollAxis;
final double ratioOfBlankToScreen;
ScrollingText({
@required this.text,
this.textStyle,
this.scrollAxis: Axis.horizontal,
this.ratioOfBlankToScreen: 0.25,
}) : assert(text != null,);
@override
State<StatefulWidget> createState() {
return ScrollingTextState();
}
}
class ScrollingTextState extends State<ScrollingText>
with SingleTickerProviderStateMixin {
ScrollController scrollController;
double screenWidth;
double screenHeight;
double position = 0.0;
Timer timer;
final double _moveDistance = 3.0;
final int _timerRest = 100;
GlobalKey _key = GlobalKey();
@override
void initState() {
super.initState();
scrollController = ScrollController();
WidgetsBinding.instance.addPostFrameCallback((callback) {
startTimer();
});
}
void startTimer() {
if (_key.currentContext != null) {
double widgetWidth =
_key.currentContext.findRenderObject().paintBounds.size.width;
double widgetHeight =
_key.currentContext.findRenderObject().paintBounds.size.height;
timer = Timer.periodic(Duration(milliseconds: _timerRest), (timer) {
double maxScrollExtent = scrollController.position.maxScrollExtent;
double pixels = scrollController.position.pixels;
if (pixels + _moveDistance >= maxScrollExtent) {
if (widget.scrollAxis == Axis.horizontal) {
position = (maxScrollExtent -
screenWidth * widget.ratioOfBlankToScreen +
widgetWidth) /
2 -
widgetWidth +
pixels -
maxScrollExtent;
} else {
position = (maxScrollExtent -
screenHeight * widget.ratioOfBlankToScreen +
widgetHeight) /
2 -
widgetHeight +
pixels -
maxScrollExtent;
}
scrollController.jumpTo(position);
}
position += _moveDistance;
scrollController.animateTo(position,
duration: Duration(milliseconds: _timerRest), curve: Curves.linear);
});
}
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
screenWidth = MediaQuery.of(context).size.width;
screenHeight = MediaQuery.of(context).size.height;
}
Widget getBothEndsChild() {
if (widget.scrollAxis == Axis.vertical) {
String newString = widget.text.split("").join("\n");
return Center(
child: Text(
newString,
style: widget.textStyle,
textAlign: TextAlign.center,
),
);
}
return Center(
child: Text(
widget.text,
style: widget.textStyle,
));
}
Widget getCenterChild() {
if (widget.scrollAxis == Axis.horizontal) {
return Container(width: screenWidth * widget.ratioOfBlankToScreen);
} else {
return Container(height: screenHeight * widget.ratioOfBlankToScreen);
}
}
@override
void dispose() {
super.dispose();
if (timer != null) {
timer.cancel();
}
}
@override
Widget build(BuildContext context) {
return ListView(
key: _key,
scrollDirection: widget.scrollAxis,
controller: scrollController,
physics: NeverScrollableScrollPhysics(),
children: <Widget>[
getBothEndsChild(),
getCenterChild(),
getBothEndsChild(),
],
);
}
}
And use the widget like this:
ScrollingText(
text: text,
textStyle: TextStyle(fontSize: 12),
)
Solution 2
use this widget from pub.dev marquee.
Marquee(
text: 'There once was a boy who told this story about a boy: "',
)
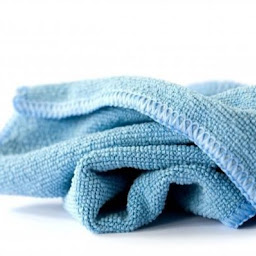
Author by
Butzlabben
Updated on December 10, 2022Comments
-
Butzlabben over 1 year
I am building a radio app. Like in Spotify, there is a bar with the current title and artist, the text should be in one line and in a given width. How can I let the text move from right to left and back?
When using a self-made animation, I want to have a fixed speed of the moving text, so I need the time and the width of the text widget.
Is there a package/built-in option to do this? Or do I have to use a self-made animation? If so, how can I get the text widget width?
Controller and animation:
AnimationController(duration: Duration(seconds: 10), vsync: this); animation = Tween<double>(begin: 0, end: 1) .animate(CurvedAnimation(parent: _controller, curve: Curves.linear)); animation.addListener(() { setState(() {}); }); _controller.repeat();
build method
double value = -300 * (animation.value <= 0.5 ? animation.value : 1 - animation.value); return Container( child: SizedBox( width: widget.width, height: 24, child: Transform.translate( offset: Offset(value, 0), child: widget.text, ), ), );