How I can convert a bitmap into PDF format in android
18,129
Solution 1
you can do by this way...you have to download itextpdf-5.3.2.jar file and attach in your project..
public class WritePdfActivity extends Activity
{
private static String FILE = "mnt/sdcard/FirstPdf.pdf";
static Image image;
static ImageView img;
Bitmap bmp;
static Bitmap bt;
static byte[] bArray;
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
img=(ImageView)findViewById(R.id.imageView1);
try
{
Document document = new Document();
PdfWriter.getInstance(document, new FileOutputStream(FILE));
document.open();
addImage(document);
document.close();
}
catch (Exception e)
{
e.printStackTrace();
}
}
private static void addImage(Document document)
{
try
{
image = Image.getInstance(bArray); ///Here i set byte array..you can do bitmap to byte array and set in image...
}
catch (BadElementException e)
{
// TODO Auto-generated catch block
e.printStackTrace();
}
catch (MalformedURLException e)
{
// TODO Auto-generated catch block
e.printStackTrace();
}
catch (IOException e)
{
// TODO Auto-generated catch block
e.printStackTrace();
}
// image.scaleAbsolute(150f, 150f);
try
{
document.add(image);
} catch (DocumentException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
Solution 2
You need to use a 3rd party library, there's no built in ability. I know a few libraries that do the reverse (Qoppa, PDFTron, Reade) but they all cost a lot of money. I've heard iText works well for writing to bitmaps, but haven't used it myself.
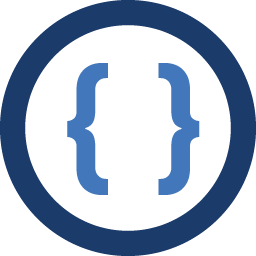
Author by
Admin
Updated on June 15, 2022Comments
-
Admin almost 2 years
**I have bitmap in "thepic" variable which is of Bitmap type..
imageUri = (Uri) intent.getParcelableExtra(Intent.EXTRA_STREAM); String realpath=getRealPathFromURI(imageUri); thepic = BitmapFactory.decodeFile(realpath);**
-
baldguy over 11 years@VarunSingh Whether this helps you or not. Please notify me.
-
JoxTraex over 11 yearsIf you're going to recommend something, please atleast mention what library you are using. That is not in the standard android SDK. @Mehul
-
Mehul Ranpara over 11 years@JoxTraex..you right..i forgot to mention the itextpdf-5.3.2.jar file.
-
Admin over 11 yearsThanx For help. Its working But Problem is that when I click on Convert to PDF the file is saved on specified path "mnt/sdcard/FirstPdf.pdf"; and the old pdf file is replaced with new one as every time I convert an image it is saved as FirstPdf.pdf.
-
Mehul Ranpara over 11 yearsyou can give any name..inplace of Firstpdf.pdf to img1.pdf/img2.pdf
-
Admin over 11 years@MehulRanpara Actually I'm capturing that image from camera and then click on Convert to pdf button. So Every time there is a new image and if I give any name then every image is saved with that name replacing previous.
-
Admin over 11 years@MehulRanpara I have solved my problem. Thank you...
-
Mehul Ranpara over 11 yearsWelcome.. Bro..if you satisfy then upvote my answer
-
Admin over 11 years@MehulRanpara Actually voting up requires min. 15 reputation and I have made a/c on stack overflow 2 days ago so currently I have 8 reputation. When I will have more than 15 I will vote up.
-
Snehal Poyrekar over 10 years@MehulRanpara is this jar free or does it require a fee if i have to use it for commercial purpose..any idea?
-
Mehul Ranpara over 10 yearsYou can use for commercial purpose..you have not to pay anything for this jar file..
-
kangear about 10 yearsit's ok build in eclipse,but not ok build in Android source.i get this: ImageService.java:167: cannot access java.awt.Image class file for java.awt.Image not found image = Image.getInstance(bArray); // /Here i set byte array..you
-
Salman Khan over 9 years@MehulRanpara, I have implemented in the same way as you suggested. The thing is that when I am creating Bitmap and setting it to an Imageview its getting full image of all the contents present in my ScrollView. But while generating PDF for the same bitmap its showing only some part of the full image. Can you help me to sort out this.
-
TBieniek over 8 years@MehulRanpara iTextPDF can not be used for commercial purposes unless you buy a commercial license. This library is otherwise published under the AGPL copyleft license so anyone using it must publish their source code too! (see github.com/itext/itextpdf/blob/master/LICENSE.md)
-
Jeetu over 8 yearshello @SalmanKhan i have same problem i am also unable to store pdf of full bitmap have you solved your problem?