How to add a polygon on google maps?
Well, if you can convert the coordinates of your Position
objects to LatLng
objects, then you can use google_maps_flutter itself to draw polygons. You can see that one of its parameters allows for Polygons. So just building upon the example from google_maps_flutter, we'll first setup our polygon:
Set<Polygon> myPolygon() {
List<LatLng> polygonCoords = new List();
polygonCoords.add(LatLng(37.43296265331129, -122.08832357078792));
polygonCoords.add(LatLng(37.43006265331129, -122.08832357078792));
polygonCoords.add(LatLng(37.43006265331129, -122.08332357078792));
polygonCoords.add(LatLng(37.43296265331129, -122.08832357078792));
Set<Polygon> polygonSet = new Set();
polygonSet.add(Polygon(
polygonId: PolygonId('test'),
points: polygonCoords,
strokeColor: Colors.red));
return polygonSet;
}
Then just provide it to the GoogleMap widget.
GoogleMap(
polygons: myPolygon(),
mapType: MapType.normal,
initialCameraPosition: _kGooglePlex,
onMapCreated: (GoogleMapController controller) {
_controller.complete(controller);
},
),
This will draw a small triangle near the GooglePlex.
For more information on this feature, you can refer to the Android documentation of Maps SDK Shapes. The API usage should be same, if not similar, so you can use it as a guideline.
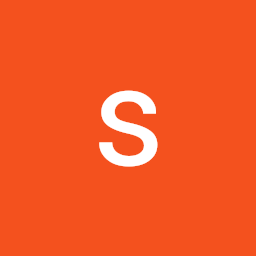
sejal.8
Updated on December 12, 2022Comments
-
sejal.8 over 1 year
I'm using google_maps_flutter plugin to use google maps. I want to draw a polygon on google maps with coordinates taken from the user. The coordinates taken are type of 'Position' object.
I have already tried using map_view plugin to draw polygons. But it didn't work for me.
This is the code snippet where the coordinates are being taken.
Geolocator geolocator = Geolocator(); StreamSubscription<Position> _positionStreamSubscription; final List<Position> _positions = <Position>[]; void _toggleListening() { if (_positionStreamSubscription == null) { const LocationOptions locationOptions = LocationOptions(accuracy: LocationAccuracy.best, distanceFilter: 10); final Stream<Position> positionStream = Geolocator().getPositionStream(locationOptions); _positionStreamSubscription = positionStream.listen( (Position position) => setState(() => _positions.add(position))); _positionStreamSubscription.pause(); } setState(() { if (_positionStreamSubscription.isPaused) { _positionStreamSubscription.resume(); } else { _positionStreamSubscription.pause(); } });