How to add an item to a list in Kotlin?
Solution 1
A more idiomatic approach would be to use MutableList
instead of specifically ArrayList
. You can declare:
val listOfVehicleNames: MutableList<String> = mutableListOf()
And add to it that way. Alternatively, you may wish to prefer immutability, and declare it as:
var listOfVehicleNames: List<String> = emptyList()
And in your completion block, simply reassign it:
listOfVehicleNames = response.body()?.message()?.orEmpty()
.map { it.name() /* assumes name() function exists */ }
Solution 2
Talking about an idiomatic approach... 🙄
When you can get away with only using immutable lists (which means usually in Kotlin), simply use +
or plus
. It returns a new list
with all elements of the original list plus the newly added one:
val original = listOf("orange", "apple")
val modified = original + "lemon" // [orange, apple, lemon]
original.plus("lemon")
yields the same result as original + "lemon"
. Slightly more verbose but might come in handy when combining several collection operations:
return getFruit()
.plus("lemon")
.distinct()
Besides adding a single element, you can use plus
to concatenate a whole collection too:
val original = listOf("orange", "apple")
val other = listOf("banana", "strawberry")
val newList = original + other // [orange, apple, banana, strawberry]
Disclaimer: this doesn't directly answer OP's question, but I feel that in a question titled "How to add an item to a list in Kotlin?", which is a top Google hit for this topic, plus
must be mentioned.
Solution 3
If you don't want or can't use array list directly use this code for add item
itemsList.toMutableList().add(item)
itemlist : list of your items
item : item you want to add
Solution 4
instead of using a regular list which is immutable just use an arrayListof which is mutable
so your regular list will become
var listofVehicleNames = arrayListOf("list items here")
then you can use the add function
listOfVehicleNames.add("what you want to add")
Solution 5
you should use a MutableList like ArrayList
var listofVechileName:List<String>?=null
becomes
var listofVechileName:ArrayList<String>?=null
and with that you can use the method add
https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-mutable-list/add.html
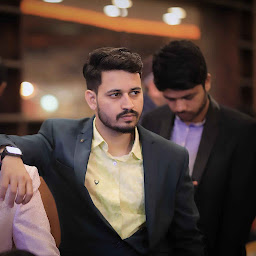
Mohit Lakhanpal
Updated on July 05, 2022Comments
-
Mohit Lakhanpal almost 2 years
I'm trying to add an element list to the list of string, but I found
Kotlin
does not have an add function likejava
so please help me out how to add the items to the list.class RetrofitKotlin : AppCompatActivity() { var listofVechile:List<Message>?=null var listofVechileName:List<String>?=null var listview:ListView?=null var progressBar:ProgressBar?=null override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_retrofit_kotlin) listview=findViewById<ListView>(R.id.mlist) var apiInterfacee=ApiClass.client.create(ApiInterfacee::class.java) val call=apiInterfacee.getTaxiType() call.enqueue(object : Callback<TaxiTypeResponse> { override fun onResponse(call: Call<TaxiTypeResponse>, response: Response<TaxiTypeResponse>) { listofVechile=response.body()?.message!! println("Sixze is here listofVechile ${listofVechile!!.size}") if (listofVechile!=null) { for (i in 0..listofVechile!!.size-1) { //how to add the name only listofVechileName list } } //println("Sixze is here ${listofVechileName!!.size}") val arrayadapter=ArrayAdapter<String>(this@RetrofitKotlin,android.R.layout.simple_expandable_list_item_1,listofVechileName) listview!!.adapter=arrayadapter } override fun onFailure(call: Call<TaxiTypeResponse>, t: Throwable) { } }) } }
-
Peppe Scab about 5 yearswhat JB Nizet said is true, but if my answer solved your question please mark it as correct
-
Peppe Scab about 5 yearsI didnt want to change too much author, but as someone makes me notice you should avoid to init to null, it would be better using lazeinit
-
SMBiggs over 4 yearsOh yeah, that's so obvious! Much easier than java. I'm so glad we switched.
-
The incredible Jan almost 3 years@SMBiggs You mean that ironically, don't you?
-
The incredible Jan almost 3 yearsDidn't you miss an assignment? toMutableList() creates a copy of itemsList and doesn't change it, right?
-
SMBiggs over 2 years@TheincredibleJan Yes. To quote TJ Miller, "The problem with satire is that it's so easily misinterpreted."
-
jure over 2 yearsI think you're correct, an assignment is missing and also, .add(item) returns a Boolean so this has to be done in multiple steps, if I'm not mistaken.
-
Jay Whitsitt almost 2 yearsThe one thing with
.plus()
is that it has to be a MutableList. Regular List doesn't implement that. -
Jonik almost 2 years@JayWhitsitt That is incorrect. Please try running the code examples in my answer. For example
listOf
returns a read-only list, yet you absolutely can callplus()
on that. As I mentioned above,plus
returns a new list; it never modifies the original list.