How to add users from data in a text file
Solution 1
Lets say your file is named just file
. This script will do the job:
USERNAME=$(cat file | cut -d: -f1)
echo "$USERNAME"
ID=$(cat file | cut -d: -f2)
echo "$ID"
USER_SHELL=$(cat file | cut -d, -f2 | cut -d: -f2)
echo "$USER_SHELL"
useradd -m -s "$USER_SHELL" -u "$ID" "$USERNAME"
Solution 2
This is a bare minimum script to get the job done. It makes sure that neither the username nor the uid is already in use. It makes a matching group for each user (with gid=uid) - it doesn't check if the gid or group name already exists (left as an exercise for the reader - hint: use getent group
).
Note: the script below is untested but I've written scripts a lot like it a million times before (slight exaggeration)....there may be some minor bugs that need fixing.
#! /bin/bash
# get newusers file from first arg on cmd line or default to 'newusers.txt'
nf="${1:-newusers.txt}"
# get existing usernames and uids.
names="^($(getent passwd | cut -d: -f1 | paste -sd'|'))$"
uids="^($(getent passwd | cut -d: -f3 | paste -sd'|'))$"
yesterday=$(date -d yesterday +%Y-%m-%d)
# temp file for passwords
tf=$(mktemp) ; chmod 600 "$tf"
while IFS=: read u uid gecos shell; do
gid="$uid" ; homedir="/home/$u"
useradd -e "$yesterday" -m -d "$homedir" -c "$gecos" \
-u "$uid" -g "$gid" -s "$shell" "$u"
groupadd -g "$gid" "$u"
# generate a random password for each user..
p=$(makepasswd)
echo "$u:$p" >> "$tf"
done < <(awk -F: '$1 !~ names && $2 !~ uids' names="$names" uids="$uids" "$nf")
# uncomment to warn about users not created:
#echo Users not created because the username or uid already existed: >&2
#awk -F: '$1 ~ names || $2 ~ uids' names="$names" uids="$uids" "$nf" >&2
# uncomment the cat to display the passwords in the terminal
echo ; echo "passwords are saved in $tf"
# cat "$tf"
# set passwords using `chpasswd:
chpasswd < "$tf"
Use pwgen
or makepassword
or any similar program if makepasswd
is not installed. Or write your own that concatenates 4+ random 5+ letter words to get an easy to remember password at least 20 characters long - capitalise some words and insert random 1-3 digit numbers and or a punctuation symbol between each word to make the password even longer and increase the brute-force search space. Random password generation is something that has been re-invented many times.
You can print out the usernames and passwords (from "$tf"
) and cut them into strips (leave a few blank lines between each user:password
) to give to each user. Tell them to change their password immediately and destroy the strip of paper. The passwords are set to expire "$yesterday"
(requires GNU date
), so the users should be prompted to change them the first time they login to their shell.
Related videos on Youtube
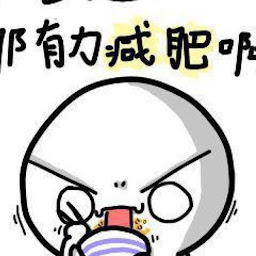
pete
Updated on September 18, 2022Comments
-
pete over 1 year
I have a question about creating multiple users in linux, but I need a program to work on it. There is a new users txt file, the content is following:
adams:5000:Adams, John Couch:/bin/bash atiyah:5001:Atiyah, Michael:/bin/csh babbage:5002:Babbage, Charles:/bin/csh baker:5003:Baker, Alan:/bin/csh barrow:5004:Barrow, Isaac:/bin/bash
... (there are 70 users name in the file)
I would like to know how to write a script to add those users automatically.
-
John almost 8 yearsWhat have you tried? We aren't going to write it for you, just help you figure out what is wrong with the script you are writing.
-
Julie Pelletier almost 8 yearsYour input is really close to a password file entry, but you could use your distribution's built-in
useradd
(or similar) command with parameters extracted from the input.
-
-
Alessio almost 8 yearsdon't use backticks. use
$()
instead. -
Alessio almost 8 yearsit would probably be better to do this in
perl
...that's what i usually use for tasks like this.