How to add values to Firebase Firestore without overwriting?
Solution 1
I suggest you to add one more document or collection that it will be able to store more just one data values for single user.
You can create a document references for both activities:
firebaseFirestore.collection("Users").document(user_id+"/acitivity1").set(data);
//and
firebaseFirestore.collection("Users").document(user_id+"/acitivity2").set(data);
Or you can create a sub-collection for it:
firebaseFirestore.collection("Users").document(user_id)
.collection("Activities").document("acitivity1").set(data);
//and
firebaseFirestore.collection("Users").document(user_id)
.collection("Activities").document("acitivity2").set(data);
More about hierarchical data there.
Solution 2
There are two ways in which you can achieve this. First one would be to use a Map
:
Map<String, Object> map = new HashMap<>();
map.put("yourProperty", "yourValue");
firebaseFirestore.collection("Users").document(user_id).update(map);
As you can see, I have used update()
method instead of set()
method.
The second approach would be to use an object of your model class like this:
YourModelClass yourModelClass = new YourModelClass();
yourModelClass.setProperty("yourValue");
firebaseFirestore.collection("Users").document(user_id)
.set(yourModelClass, SetOptions.mergeFields("yourProperty"));
As you can see, I have used the set()
method but I have passed as the second argument SetOptions.mergeFields("yourProperty")
, which means that we do an update only on a specific field.
Solution 3
If you know that the user document allready exists in firestore then you should use
firebaseFirestore.collection("Users").document(user_id).update(data)
If you don't know if the document exists then you can use
firebaseFirestore.collection("Users").document(user_id).set(data, {merge:true})
This performs a deep merge of the data
Alternatively you can do it by using subcollections
Solution 4
As per the documentation, you could use as a second parameter {merge:true}
, in my experience the problem usually is in the fact that you are trying to store different data but with the same key.
Even using {merge: true}
will always update the current key with the value you are passing in.
Merge:true Works only if the key does not exist already. I believe every key in a document must be unique.
To test it try to pass(keeping {merge: true}
as the second parameter) data with a different key, it will merge to existing.
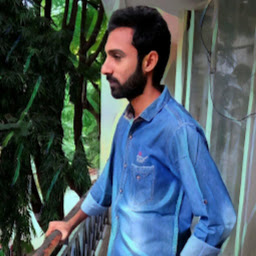
Thrinadh Reddy
Updated on June 05, 2022Comments
-
Thrinadh Reddy about 2 years
I have two Activities, I am adding data to Firestore from these two activities individually. But, whenever I add second activity data to Firestore, it is overwriting the first activity data. I used below code in the two activities:
firebaseFirestore.collection("Users").document(user_id).set(data)
How to stop overwriting? I want to save both Activities data in the same
user_id
. -
Alex Mamo over 6 yearsHave you achieved to update a value without overwriting?
-
Thrinadh Reddy over 6 years....I got it,, But i faced another problem a little later. I used a ScrollView in my Layout. But it is not scrolling the full content. After scrolling, it still leaves some scrollable portion at the bottom. And it formed small unusual white space at the bottom of the screen. how can i solve it
-
Alex Mamo over 6 yearsThis is basically another question. In order to follow the rules of this comunity, please post another fresh question, so me and other users can help you.
-
Alex Mamo over 6 yearsDid my answer helped you?
-
Thrinadh Reddy over 6 yearsI am a beginner in android programming... I don't even know how to use Model classes. So, I have not tried it. But, I liked the answer you posted for my previous question about Switch statement and Chechboxes usage. It was very helpful. That is why I asked you another question in the comments section.
-
Alex Mamo over 6 yearsYou're beginner, I understand but that is how you solved your actual problem, by adding a new document/collection? I'm happy to hear that the problem regarding the switch statement helped you.
-
Thrinadh Reddy over 6 yearsYes, I added new Document and Collection in two activities. it worked
-
Alex Mamo over 6 yearsIn this case, your question is wrong. You have asked how
to stop overriding
in terms of updating a document but you ended up solving this problem by creating new documents. Is that realy what you wanted? -
Thrinadh Reddy over 6 yearsI want to add two Activities results under one Document. Of course, I did not achieve what i wanted. Anyway, I ended up creating separate documents for both. I settled for the less.
-
Columb1a almost 4 yearsIf somebody needs to know about following scenarios: First example works perfect if the field already exist. Second example works perfect if the field doesn't exist yet.
-
Nadeem Shaikh almost 4 yearsbut what if "yourProperty" mentioned here is a arraylist and I only want to append to it?
-
Alex Mamo almost 4 years@NadeemShaikh In that case, please check the docs regarding update elements in an array.
-
Nadeem Shaikh almost 4 yearsI have gone through that. But my question is what will happen if I directly call the update function as mentioned over there without checking document exists or not?
-
Alex Mamo almost 4 years@NadeemShaikh I think this answer might help.