How to align text in a button in flutter?
42,711
Solution 1
Put it in an inkwell
InkWell(
onTap: doSomething,
child: SizedBox(
height: 100,
width: 100,
child: Container(
decoration: BoxDecoration(
border: Border.all(color: Colors.black)),
child: Text(
'hello',
textAlign: TextAlign.right,
),
),
),
),
Solution 2
You should put your 'Text' in 'Align' to align your text left, right, top, bottom etc. As-
FlatButton(
color: Colors.blue,
textColor: Colors.white,
padding: EdgeInsets.all(8.0),
splashColor: Colors.blueAccent,
onPressed: () {
/*...*/
},
child: Align(
alignment: Alignment.center,
child: Text(
"Flat",
style: TextStyle(fontSize: 20.0),
textAlign: TextAlign.center
),
))
Solution 3
It works for me
ButtonTheme(
child: FlatButton(
padding: EdgeInsets.all(0),
child: Align(
alignment: Alignment.centerLeft,
child: Text(
"Button text",
style: TextStyle(
color: ThemeColors.primaryDark,
fontWeight: FontWeight.normal,
fontSize: ThemeSizes.normalFont,
),
textAlign: TextAlign.left,
),
),
onPressed: () => _doSomething(),
),
)
Solution 4
For the new Buttons and Button Themes:
TextButton(
onPressed: () => Navigator.pop(context),
child: Text(
'Cancel',
style: Theme.of(context).typography.black.bodyText2,
),
style: ButtonStyle(
alignment: Alignment.centerLeft, // <-- had to set alignment
padding: MaterialStateProperty.all<EdgeInsetsGeometry>(
const EdgeInsets.all(0), // <-- had to set padding to 0
),
),
),
Solution 5
I found that using the padding values in the FlatButton widget worked very well.
See example below...
FlatButton(
onPressed: () {
/*...*/
},
padding: EdgeInsets.only(right: 10.0, bottom: 1.0, top: 1.0),
child: Text(
'Getting Started',
style: TextStyle(
color: Colors.blueGrey[900],
fontSize: 12.0
), //TextStyle
), //Text
), //FlatButton
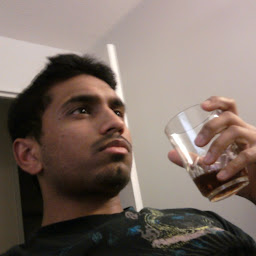
Author by
Alex Joseph
Updated on January 13, 2022Comments
-
Alex Joseph over 2 years
I just want to do the most simplest of things: I just want to have a button with the text aligned to the right. For some reason I can't figure this out.
I tried textAlign: TextAlign.right but it's still in the centre. I tried making a row inside the button with mainAxisAlignment.end but nope, still in center. for some reason it works if I use main axis alignment.end on a column instead of a row (puts it at bottom instead of right)
This is what I have so far:
FlatButton( color: Colors.black, child: Row( mainAxisAlignment: MainAxisAlignment.end, children: <Widget>[ Text( "M", textAlign: TextAlign.right, style: TextStyle( color: mColor, fontSize: 10.0), ), ], ),
-
Alex Joseph almost 5 years
-
Alex Joseph almost 5 yearsThis worked thanks! Also, I figured out you can use stack to overlay a button on top of a text widget but this is neater.
-
TSlegaitis about 4 yearsEmm, thats terrible idea :) Sorry. If text changes and button has fixed width or full screen width you'll run into big problems and you wont even know it :) using padding will only work if button does not have set width of any kind
-
rehman_00001 over 3 yearsThanks! This should be the accepted answer!
-
Son Nguyen almost 3 yearsFlatButton and ButtonTheme are not used anymore, use the new TextButton and TextButtonTheme instead. See breaking changes here: flutter.dev/docs/release/breaking-changes/buttons
-
Matteo Toma about 2 yearsThanks , doing this child: Align( alignment: Alignment.centerLeft, child: Text( worked for me