How to bind spacebar key to a certain method in tkinter (python)
Solution 1
from Tkinter import *
from random import *
root=Tk()
canvas=Canvas(root,width=400,height=300,bg='white')
def draw(event=None):
canvas.delete(ALL)# clear canvas first
canvas.create_oval(randint(0,399),randint(0,299),15,15,fill='red')
draw()
canvas.pack()
root.bind("<space>", draw)
root.mainloop()
Solution 2
You could do something like this:
from Tkinter import *
from random import *
root=Tk()
canvas=Canvas(root,width=400,height=300,bg='white')
def draw(event):
if event.char == ' ':
canvas.delete(ALL)# clear canvas first
canvas.create_oval(randint(0,399),randint(0,299),15,15,fill='red')
root.bind('<Key>', draw)
canvas.pack()
root.mainloop()
Basically, you bind your drawing function to some top-level element to the <Key>
binding which is triggered whenever a key on the keyboard is pressed. Then, the event object that's passed in has a char
member which contains a string representing the key that was pressed on the keyboard.
The event will only be triggered when the object it's bound to has focus, which is why I'm binding the draw
method to the root
object, since that'll always be in focus.
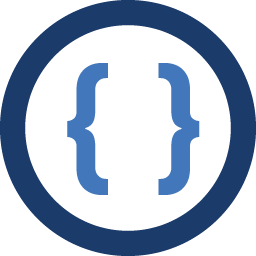
Admin
Updated on November 28, 2020Comments
-
Admin over 3 years
I am working on a project in python, and I made a method to draw a specific thing in tkinter. I want it so that whenever I press the spacebar, the image will redraw itself (run the method again because I coded the method so that it could redraw over itself). How exactly would I bind the spacebar to the method so that the program would run, draw, and re-draw if I pressed the spacebar?
for example, i want it so that whenever I press space, the program draws in a random location on the canvas:
from Tkinter import * from random import * root=Tk() canvas=Canvas(root,width=400,height=300,bg='white') def draw(): canvas.delete(ALL)# clear canvas first canvas.create_oval(randint(0,399),randint(0,299),15,15,fill='red') draw() canvas.pack() root.mainloop()
how would i bind the spacebar to the method?