How to calculate distance between two cities using google maps api V3
43,528
What you want is the Distance Matrix API. That is, if you are really using it in combination google maps. Note that Google prohibits the use of this API if you are not using it with a Google Map somewhere on the page.
Here's a basic example of the Distance Matrix API:
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript" src="http://maps.google.com/maps/api/js?sensor=false"></script>
<script type="text/javascript">
var origin = new google.maps.LatLng(55.930385, -3.118425),
destination = "Stockholm, Sweden",
service = new google.maps.DistanceMatrixService();
service.getDistanceMatrix(
{
origins: [origin],
destinations: [destination],
travelMode: google.maps.TravelMode.DRIVING,
avoidHighways: false,
avoidTolls: false
},
callback
);
function callback(response, status) {
var orig = document.getElementById("orig"),
dest = document.getElementById("dest"),
dist = document.getElementById("dist");
if(status=="OK") {
orig.value = response.destinationAddresses[0];
dest.value = response.originAddresses[0];
dist.value = response.rows[0].elements[0].distance.text;
} else {
alert("Error: " + status);
}
}
</script>
</head>
<body>
<br>
Basic example for using the Distance Matrix.<br><br>
Origin: <input id="orig" type="text" style="width:35em"><br><br>
Destination: <input id="dest" type="text" style="width:35em"><br><br>
Distance: <input id="dist" type="text" style="width:35em">
</body>
</html>
This is a version of google's example adapted to your personal taste which I found in the Google Maps Api v3 - Distance Matrix section
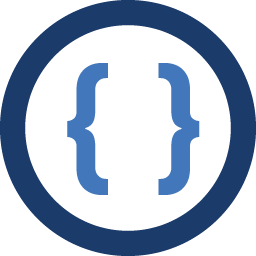
Author by
Admin
Updated on July 28, 2020Comments
-
Admin almost 4 years
How to calculate the driving distance ?
I'm unable to find a working example, can anybody help me ?
Thank you.
EDIT: More info:
I have two text inputs to type the cities. On change I just want to display/update the distance in another div.