How to cast a string to a function pointer in C?
Solution 1
Actually, if the functions are extern
, can you not do this with some super-duper evil dynamic loader trickery?
Solution 2
Read the duplicate, you'll find your answer.
I wanted to add, though, that this comes from a misunderstanding of how the program works.
These functions are at addresses in memory. Strings are not addresses. The names of functions, in code, are synonymous with their address. At runtime, having a string is really just having a pointer to some place in memory with characters. This has no relation to anything done at compile time, like function names.
Some languages include meta-data about the program, which means they do carry information about functions, their names, parameters, etc... at run time. C++ is not one of these languages.
This is why most answers will be a sort of simulation of this meta data: functions will be stored, and at run time you can use the string to peek into the table.
Solution 3
You can't turn strings into function pointers in C. You have to check the contents of the string to determine which function to use.
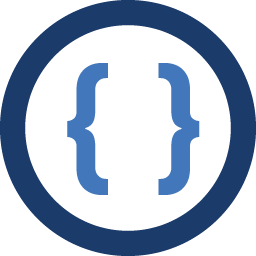
Admin
Updated on June 05, 2022Comments
-
Admin about 2 years
Possible Duplicates:
call a function named in a string variable in c
Dynamically invoking any function by passing function name as stringI have certain functions.. say,
double fA1B1C1( .. ) { ... } double fA2B2C3( .. ) { ... } double (*fptr)( .. ); fptr myfunc;
These functions are already defined.
So when the user inputs the string "A2B2C2", it is got into a variable and converted to "fA2B2C2"
myfunc = formatted_string; // DOES NOT WORK
But the above syntax does not work. Even typecasting it does not work.
myfunc = (fptr) formatted_string // DOES NOT WORK
But if I hard code it as
myfunc = fA2B2C2
(or)myfunc = &fA2B2C2
, it works pefectly.Where am I going wrong?
EDIT::
I tried creating a hash table and a lookup function. Like create_hashEntry(hashtable, string, formatted_string); // string = "A1B1C1; formatted_string = "fA1B1C1"
then the look up function
myfunc lookup_func(char *string) { return(get_hashEntry(hahstable, string)); }
This also failed to work.
-
GManNickG almost 15 yearsDuplicate: stackoverflow.com/questions/1118705/…
-
Admin almost 15 yearsYou are mistaking C for a scripting language.
-
dave4420 almost 15 yearsYou have added the (name, function) pairs to the hash table, yes? (And before you run the lookup function, too?) Your code doesn't show this, only a comment.
-
ezpz almost 15 yearsIf you are merely hashing a string to another string you arent going to solve any problems associated with converting a string to a memory location related to a function. Read the duplicate; pay special attention to the methods available for loading a library and using its symbol table to map a string to a function pointer. There are well developed interfaces for this on most popular platforms.
-
-
Vilius Surblys almost 15 years...and if you could, it would be a stupidly large security hole.
-
Admin almost 15 yearsPlease look at my edited post..
-
EFraim almost 15 yearsActually the standard does not even guarantee the ability to perform the cast, AFAIK. (i.e. the pointers can be of different sizes)