How to change background color of react native button
Solution 1
Use this for adding the background color to the button in iOS:
Styles:
loginScreenButton:{
marginRight:40,
marginLeft:40,
marginTop:10,
paddingTop:10,
paddingBottom:10,
backgroundColor:'#1E6738',
borderRadius:10,
borderWidth: 1,
borderColor: '#fff'
},
loginText:{
color:'#fff',
textAlign:'center',
paddingLeft : 10,
paddingRight : 10
}
Button:
<TouchableOpacity
style={styles.loginScreenButton}
onPress={() => navigate('HomeScreen')}
underlayColor='#fff'>
<Text style={styles.loginText}>Login</Text>
</TouchableOpacity>
For Android it simply works with Button color property only:
<Button onPress={onPressAction} title="Login" color="#1E6738" accessibilityLabel="Learn more about this button" />
Solution 2
I suggest to use React Native Elements package, which allow to insert styles throught buttonStyle
property.
styles:
const styles = StyleSheet.create({
container: {
flex: 1
},
button: {
backgroundColor: '#00aeef',
borderColor: 'red',
borderWidth: 5,
borderRadius: 15
}
})
render()
render() {
return (
<View style={styles.container}>
<Button
buttonStyle={styles.button}
title="Example"
onPress={() => {}}/>
</View>
)
}
Expo Snack: https://snack.expo.io/Bk3zI0u0G
Solution 3
"color" property applies to background only if you're building for android.
Other than that I personally find it easier to manage custom buttons. That is create your own component named button and have it as a view with text. This way its way more manageable and you can import it as easy as Button.
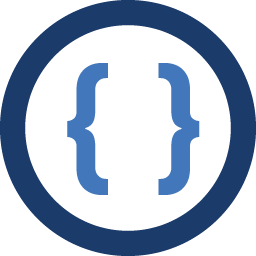
Admin
Updated on October 14, 2021Comments
-
Admin over 2 years
I am trying to change the background color of my button but nothing seems to work, I tried the raised property, maybe i am using it incorrectly. Do any of you have any ideas?
import React from 'react'; import { StyleSheet, Text, View, Button, TouchableHighlight } from 'react-native'; export default class App extends React.Component { state={ name: "Mamadou" }; myPress = () => { this.setState({ name: "Coulibaly" }); }; render() { return ( <View style={styles.container}> <Button title={this.state.name} color="red" onPress={this.myPress} /> </View> ); } } const styles = StyleSheet.create({ container: { flex: 1, backgroundColor: '#fff', alignItems: 'center', justifyContent: 'center', }, });