How to change the ImageView source dynamically from a string? (Xamarin Android)
Solution 1
Here is the standard Android method:
int resImage = getResources().getIdentifier(imgName , "drawable", getPackageName());
myTextView.setImageResource(resImage);
Please note that imgName variable must not contain file extension.
Xamarin.Android
C#:
int resImage = Resources.GetIdentifier(imgName, "drawable", PackageName);
Solution 2
Thanks to SushiHangover and Romuald for their answer, but I'd like to share another way that I tested and it looks working well.
Here's how I get the id of the resource:
int resourceId = (int)typeof(Resource.Drawable).GetField("myImageName").GetValue(null);
So, then I'm able to use the normal SetImageResource():
imgViewCondition.SetImageResource(resourceId);
Solution 3
The following code snippet solves the issue:
int id = getResources().getIdentifier("XXX", "drawable", getPackageName());
imageView.setImageResource(id);
Solution 4
Just this:
FindViewById<ImageView>(Resource.Id.imageviewName).SetImageResource(Resource.Drawable.nameofimage);
Solution 5
I know it's an old question, but it never hurt to help.
The image should be stored in all the proper sizes for android inside the Resources Folder (drawable-hdpi, drawable-ldpi ....)
and then you get it dynamically like so
imageView.SetImageResource(Resource.Drawable.YourImageNameHere);
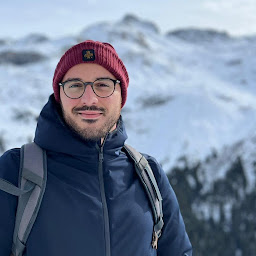
Masciuniria
Updated on June 05, 2022Comments
-
Masciuniria almost 2 years
I'm using Xamarin Android and I'm trying to change the ImageView src dynamically. All the pictures are placed in Resource/drawable. After reading the picture name as string from an xml (
string imgName = nameFromXml + ".jpg"
) I would like to passing it to myImageView
. I guess there are two good ways:1) Get the image
(int)id
and passing it to the ImageView using SetImageResource():myTextView.SetImageResource(????????);
2) Get the Uri of the of the Image
EDIT
Unfortunately I couldn't use Eric answer for QuinDa question because there are many methods not existing on Xamarin Android, working just in Android.