How to check and replace null value in C#
29,761
Solution 1
Since you're using a datatable I assume you're not talking about NULL (or nill), but DBNull.. Change your function ConvertNullToEmptyString like this:
private String ConvertNullToEmptyString(DataTable element){
if (element.Rows[0]["Fullname"] == DBNull.Value || element.Rows[0]["Fullname"] == null) {
return "NO DATA";
} else {
return element.Rows[0]["Fullname"].ToString();
}
}
as requested: new sample;
// a list of datatables each containing 1 row, wasn't that the point of datatables
// anyway -- I think you don't have any rows, so let's try this:
private String ConvertNullToEmptyString(DataTable element)
{
if (element.Rows.Count == 0)
{
return "NO DATA";
}
if (element.Rows[0]["Fullname"] == DBNull.Value || element.Rows[0]["Fullname"] == null)
{
return "NO DATA";
}
else
{
return element.Rows[0]["Fullname"].ToString();
}
}
protected void Test()
{
List<DataTable> strList = new List<DataTable>(){
GetItem("test1"), //private DataTable GetItem1(String t)
GetItem("test2") //...
};
txtGrD_D.Text = ConvertNullToEmptyString(strList[0]);
}
Solution 2
You can use C#'s null coalescing operator ??:
string s = null;
s = s ?? "NO DATA";
// s now equals "NO DATA";
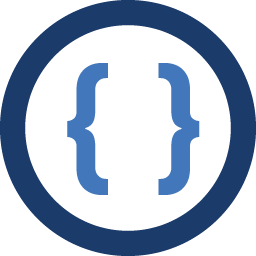
Author by
Admin
Updated on May 17, 2020Comments
-
Admin about 4 years
Anyone can guide me a way to check and replace null values in strList with String message "NO DATA" or allow them in dtList. Because when I met a null value in this List,it show an error like "There is no row at position..."
private DataTable getSomething1(String text){ DataTable dtb = new DataTable(); ... ... return dtb; } ... protected void buttonCheck_Click(object sender, ImageClickEventArgs e){ List<DataTable> strList = new List<DataTable>(){ getSomething(txtInput.Text), getSomething2(txtInput.Text), getSomething3(txtInput.Text), ... }; }
I used C# in Webform. Plz, help me to fix this code or guide me a way to solve it. Thanks in advance.
@tech, @ riffnl: Finally I get all of your ideas and it really work now. Anw, thanks all pro here for advices. So, this is my solution ^^
private String ConvertNullToEmptyString(DataTable element) { if (element.Rows.Count > 0) //just add this condition here { if (!DBNull.Value.Equals(element.Rows[0]["FullName"])) return (string)element.Rows[0]["FullName"] + " "; else return String.Empty; } return "NO DATA"; } protected void ....(){ List<DataTable> strList = new List<DataTable>(){ GetItem1(txtName.Text), //private DataTable GetItem1(String t) GetItem2(txtName.Text), //... }; txtGrD_D.Text = ConvertNullToEmptyString(strList[0]); txtGrM_D.Text = ConvertNullToEmptyString(strList[1]); }