How to check if a file has content or not using C?
Solution 1
C version:
if (NULL != fp) {
fseek (fp, 0, SEEK_END);
size = ftell(fp);
if (0 == size) {
printf("file is empty\n");
}
}
C++ version (stolen from here):
bool is_empty(std::ifstream& pFile)
{
return pFile.peek() == std::ifstream::traits_type::eof();
}
Solution 2
Just look if there's a character to read
int c = fgetc(fp);
if (c == EOF) {
/* file empty, error handling */
} else {
ungetc(c, fp);
}
Solution 3
You can do this without opening the file as well using the stat
method.
#include <sys/stat.h>
#include <errno.h>
int main(int argc, char *argv[])
{
struct stat stat_record;
if(stat(argv[1], &stat_record))
printf("%s", strerror(errno));
else if(stat_record.st_size <= 1)
printf("File is empty\n");
else {
// File is present and has data so do stuff...
}
So if the file doesn't exist you'll hit the first if
and get a message like: "No such file or directory"
If the file exists and is empty you'll get the second message "File is empty"
This functionality exists on both Linux and Windows, but on Win it's _stat
. I haven't tested the windows code yet, but you can see examples of it here.
Solution 4
fseek(fp, 0, SEEK_END); // goto end of file
if (ftell(fp) == 0)
{
//file empty
}
fseek(fp, 0, SEEK_SET); // goto begin of file
// etc;
reference for ftell and example
reference for fseek and example
Solution 5
You can use fseek using SEEK_END
and then ftell to get the size of a file in bytes.
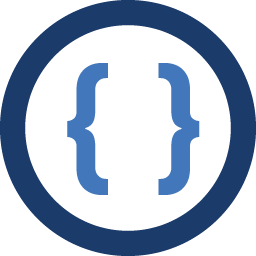
Admin
Updated on April 01, 2020Comments
-
Admin about 4 years
I have a source file
file1
and a destination filefile2
, here I have to move content fromfile1
tofile2
.So I have to do some validation first.
I must check source file is existing or not? I can check using this:
fp = fopen( argv[1],"r" ); if ( fp == NULL ) { printf( "Could not open source file\n" ); exit(1); }
Then I have to check if the source file has any content or not? If it is empty, I have to throw some error message.
This is what I've tried until the moment.