How to compress build with/without ejecting create-react-app. Also include compressed file into script tag into index.html
Solution 1
It's not really easy to change cra build process, you can eject it but you'll have to create your own after. But, in your package.json you can define a task to be launched after the build :
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject",
"postbuild": "node yourNodeGzipScript.js" }
Hope it can help.
Solution 2
Install gzipper package (https://www.npmjs.com/package/gzipper). Modify build command like this
"build": "react-scripts build && gzipper --verbose ./build"
and build your project you will get both gzip and regular file. It is upto you to make server to serve gzip instead of regular file. If you are using nginx you have to setup your server in nginx.conf file as below
server {
# Gzip Settings
gzip on;
gzip_static on; # allows pre-serving of .gz file if it exists
gzip_disable "msie6"; # Disable for user-agent Internet explorer 6. Not supported.
gzip_proxied any; # enable gzip for all proxied requests
gzip_buffers 16 8k; # number and size of buffers to compress a response
gzip_http_version 1.1;
gzip_min_length 256; # Only gzip files of size in bytes
gzip_types text/plain text/css text/html application/javascript application/json application/x-javascript text/xml application/xml application/xml+rss text/javascript application/vnd.ms-fontobject application/x-font-ttf font/opentype image/svg+xml image/x-icon;
gunzip on; # Uncompress on the fly
listen 4000;
server_name localhost;
location / {
root html/react-app;
index index.html index.htm;
}
error_page 500 502 503 504 /50x.html;
location = /50x.html {
root html;
}
}
Solution 3
You could also just add a postbuild script in your package.json with:
"postbuild": "find ./build -type f -name '*.css' -o -name '*.js' -exec gzip -k '{}' \\;"
Solution 4
I used this command to gzip files keep them the same name
- yarn global add gzipper
- gzipper compress ./build ./gzip_build --output-file-format [filename].[ext] --verbose
FYI: gzipper --verbose got me an error. Missed "compress".
Solution 5
You can add post build compression to your create-react-app build with minimal configuration using compress-create-react-app. It compresses all html, css and javascript files in the build folder using brotli and gzip algorithms.
First install it as dev dependency:
npm install compress-create-react-app --save-dev
Then edit the build command in your package.json:
"scripts": {
"start": "react-scripts start",
- "build": "react-scripts build",
+ "build": "react-scripts build && compress-cra",
"test": "react-scripts test",
"eject": "react-scripts eject"
}
That's it. Your app will be compressed when you build it.
Disclaimer: I'm the author of compress-create-react-app
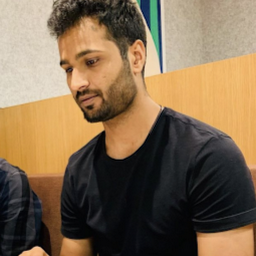
Comments
-
harish kumar almost 2 years
I'm using
react-scripts build
to make production ready build and to deploy it on AWS server I'm usingGENERATE_SOURCEMAP=false yarn build && aws s3 sync build/ s3://*********
Is there any way to apply gzip compression to build files and include gzipped files into index.html and deploy it on aws so that in browser gzipped files would be served. -
harish kumar about 5 yearsI'm able to compress the files but not able to change injected file name as .gz has to be included in the last of file name e.g changing from ** .chunks.js to ** chunks.js.gz
-
Nil about 5 yearsHave you tried using the "rename" or "copy" methods of the "fs" package?
-
harish kumar about 5 yearsYes I did that, I made a .js file and use 'fs' to update script & css tags. And it worked.. Thanks buddy.
-
harish kumar over 4 yearsI've fixed the problem from cloud front. but thanks for the solution. :)
-
Anwesh Mohapatra over 3 yearsthis should be the accepted answer. Most detailed and helpful for sure
-
Val over 2 yearsAny chance, we can run this on
npm run start
? it makes life easier, when working with lighthouse debugging in devtools. -
jnsjknn over 2 yearsI'll see what I can do but it's probably difficult to make create-react-app serve the compressed files. Feel free to make a pull request on GitHub if you have an idea on how to do it and want to contribute.
-
Amir2mi about 2 yearsYou should add
compress
to compress the command will be:react-scripts build && gzipper compress --verbose ./build
-
Sangeet Agarwal about 2 years@jnsjknn thanks! I tried your package & it seems to be working nicely. I then serve my static resources via
app.use("/", expressStaticGzip(path.resolve(__dirname)));