How to continue executing a java program after an Exception is thrown?
Solution 1
Your code should look as follows:
public class ExceptionsDemo {
public static void main(String[] args) {
for (int i=args.length;i<10;i++){
try {
if(i%2==0){
System.out.println("i =" + i);
throw new Exception(); // stuff that might throw
}
} catch (Exception e) {
System.err.println("An exception was thrown");
}
}
}
}
Solution 2
Move the try catch block within for loop and then it should work
Solution 3
You need to re-structure it slightly, so that the try/catch is inside the for loop, not enclosing it, e.g.
for (...) {
try {
// stuff that might throw
}
catch (...) {
// handle exception
}
}
As an aside, you should avoid using exceptions for flow control like that - exceptions should be used for exceptional things.
Solution 4
Just don't throw an exception, then:
int arr[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
for (int i = 0; i < arr.length; i++) {
if (i % 2 == 0) {
System.out.println("i = " + i);
}
}
Or throw it, and catch it inside the loop, rather than outside (but I don't see the point in throwing an exception in this trivial example):
int arr[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
for (int i = 0; i < arr.length; i++) {
try {
if (i % 2 == 0) {
System.out.println("i = " + i);
throw new Exception();
}
}
catch (Exception e) {
System.err.println("An exception was thrown");
}
}
Side note: see how the code is much easier to read when it's indented properly, and contains white spaces around operators.
Solution 5
You can not do that because your array is defined within the try clause. If you want to be able to access it move it out of there. Also maybe you should somehow store which i caused the Exception in the Exception so that you can continue from it.
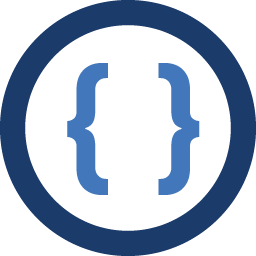
Admin
Updated on October 23, 2020Comments
-
Admin over 3 years
My sample code is as follows:
public class ExceptionsDemo { public static void main(String[] args) { try { int arr[]={1,2,3,4,5,6,7,8,9,10}; for(int i=arr.length;i<10;i++){ if(i%2==0){ System.out.println("i =" + i); throw new Exception(); } } } catch (Exception e) { System.err.println("An exception was thrown"); } } }
My requirement is that, after the exception is caught I want to process the remaining elements of the array. How can I do this?