Java: Is there an exception for when a user enters a number out of range
Solution 1
You can throw IllegalArgumentException
Solution 2
If you particularly want to use exception handling for this then you should probably define your own custom exception for it rather than reuse a standard Java one. That way you can be certain you are only catching your particular case. If you do define an exception you will need to manually throw it and catch it.
Note that I am referring here specifically to an exception related to user entry that you wish to handle. Java has an excellent set of exceptions for handling errors that are internal to your code (e.g. null pointer exceptions).
Having said that this does not seem like a good use case for an exception. Your code should consider every value a user might enter as within normal use. Exception handling is best reserved for handling things that are exceptions to normal operations.
Solution 3
Java Language Specification, regarding Integer Operations says:
The integer operators do not indicate overflow or underflow in any way.
An integer operator can throw an exception (§11) for the following reasons:
Any integer operator can throw a
NullPointerException
if unboxing conversion (§5.1.8) of a null reference is required.The integer divide operator / (§15.17.2) and the integer remainder operator % (§15.17.3) can throw an
ArithmeticException
if the right-hand operand is zero.The increment and decrement operators ++ (§15.14.2, §15.15.1) and -- (§15.14.3, §15.15.2) can throw an
OutOfMemoryError
if boxing conversion (§5.1.7) is required and there is not sufficient memory available to perform the conversion.
I m not a fan of "exception driven architecture", ;)
Implement a check if the integer is over 100 and return an error, status code, etc as feedback for user/system etc.
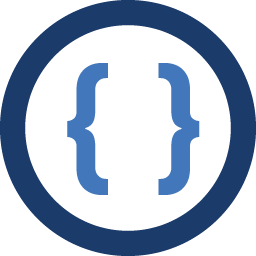
Admin
Updated on June 16, 2022Comments
-
Admin almost 2 years
Outside of the context of an Array index, is there any exception in Java that can be thrown when the user enters a number outside the range asked for by a program?
ie. The program wants an integer between 0-100 and user enters 132, could an exception be thrown manually or does it have to be custom?
EDIT: I get that it doesn't make sense to use an exception for this, it's for an assignment and I want to know my options. Easy on the hair trigger down voters.