Unhandled Exception Type in JAVA
Solution 1
You are extending Exception
, a checked exception, this means that any method which throws that exception needs to say so:
Zoo(int capacity) throws ZooException {
And any code calling that constructor will have to try {} catch {}
or throw it again.
If you don't want it to be checked, use extends RuntimeException
instead
Solution 2
About unchecked exceptions @Will P is right.
About serialVersionUID, this means - since Exception is Serializable - if you anytime decide that a previous version of your class should be incompatible with your newer version ( usually for public APIs), for example, that class had undergone major changes, simply change the unique id, and reading an object of the old version would throw an exception.
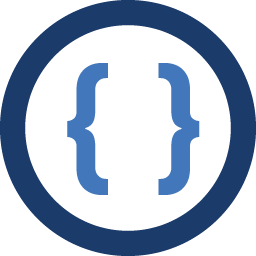
Admin
Updated on June 03, 2020Comments
-
Admin almost 4 years
I have a two classes in the same package in JAVA.
One class in which I have a constructor an exception I tried to create myself:
public class ZooException extends Exception { public ZooException(String error) { super(error); } }
Another class needs to call this exception at one point:
public class Zoo<T extends Animal> Zoo(int capacity) { if (capacity <= 1) { throw new ZooException("Zoo capacity must be larger than zero"); } }
I notice two things here
- In the ZooException class, I get a warning: "The serializable class CageException does not declare a static final serialVersionUID field of type long"
- In the Zoo class, on the line that starts with "throw new", I get a compile error: "Unhandled exception type CageException"
Any ideas on what I can do to solve this error in the Zoo class? Thank you in advance!