How to convert a char array to a byte array?
Here is a little Arduino sketch illustrating one way to do this:
void setup() {
Serial.begin(9600);
char arr[] = "abcdef98";
byte out[4];
auto getNum = [](char c){ return c > '9' ? c - 'a' + 10 : c - '0'; };
byte *ptr = out;
for(char *idx = arr ; *idx ; ++idx, ++ptr ){
*ptr = (getNum( *idx++ ) << 4) + getNum( *idx );
}
//Check converted byte values.
for( byte b : out )
Serial.println( b, HEX );
}
void loop() {
}
The loop will keep converting until it hits a null character. Also the code used in getNum
only deals with lower case values. If you need to parse uppercase values its an easy change. If you need to parse both then its only a little more code, I'll leave that for you if needed (let me know if you cannot work it out and need it).
This will output to the serial monitor the 4 byte values contained in out
after conversion.
AB
CD
EF
98
Edit: How to use different length inputs.
The loop does not care how much data there is, as long as there are an even number of inputs (two ascii chars for each byte of output) plus a single terminating null. It simply stops converting when it hits the input strings terminating null.
So to do a longer conversion in the sketch above, you only need to change the length of the output (to accommodate the longer number). I.e:
char arr[] = "abcdef9876543210";
byte out[8];
The 4
inside the loop doesn't change. It is shifting the first number into position.
For the first two inputs ("ab"
) the code first converts the 'a' to the number 10
, or hexidecimal A
. It then shifts it left 4 bits, so it resides in the upper four bits of the byte: 0A
to A0
. Then the second value B
is simply added to the number giving AB
.
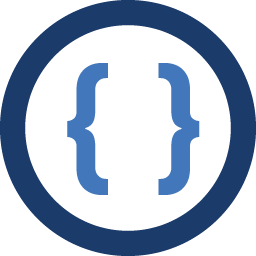
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I'm working on my project and now I'm stuck with a problem that is, how can I convert a char array to a byte array?.
For example: I need to convert
char[9]
"fff2bdf1"
to a byte array that isbyte[4]
is0xff,0xf2,0xbd,0xf1
. -
Karsten Koop almost 8 yearsCan you explain a bit more what's going on in your code? I don't understand how this parses hex values.
-
Admin almost 8 yearsThank you for your comment. I'm trying to understand your code and edit it to the case of an array of 6 bytes. I edited the number '4' to 6 but it's not work. Can you explain a little bit further about your code?.
-
Chris A almost 8 years@TrungPhạmKhánh I have edited the end of the question with an explanation.
-
MICRO almost 7 yearsHow to parse only upper case? If the
char arr[] = "ABCDEF98"
-
mehmet over 2 yearswhat is data, &data[i]