How do I convert char to int on Arduino serial read()?
Solution 1
Your issue is a bit more nuanced than you have laid out. Since Serial.read() will give you each character one at a time, if you type "180" in the serial monitor you will get '1' then '8' then '0'.
When you receive a char and change to an int you will get the char equivalent in ASCII. The value of '0' is actually 48 so you will need to handle that. Then with each successive char you will need to shift the result right by one space (power of 10) and insert the new value in the 1's column to reassemble the angle typed in.
Here is some code which should work:
#include <Servo.h>
Servo myservo;
void setup()
{
myservo.attach(9);
Serial.begin(9600);
myservo.write(0); //or another starting value
}
void loop()
{
//reset the values each loop but only reprint
//them to the servo if you hit the while loop
int angle = 0;
while(Serial.available() > 0){
//Get char in and convert to int
char a = Serial.read();
int c = (int)a - 48;
//values will come in one character at a time
//so you need to increment by a power of 10 for
//each character that is read
angle *= 10;
//then add the 1's column
angle += c;
//then write the angle inside the loop
//if you do it outside you will keep writing 0
Serial.println(angle);//TODO this is a check. comment out when you are happy
myservo.write(angle);
}
}
Solution 2
In short, in your case it is more appropriate to use Serial.parseInt()
:
void loop() {
if (Serial.available()) {
int c = Serial.parseInt();
myservo.write(c);
}
}
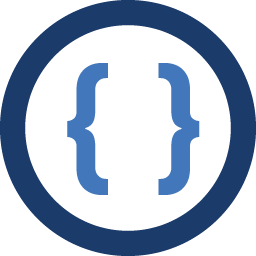
Admin
Updated on April 15, 2021Comments
-
Admin about 3 years
I send string value from my Android device to Arduino, but I can not convert input
serial.read()
to a real integer value.How can I get an integer number between 1..180 (to control a servo motor)?
void setup() { myservo.attach(9); Serial.begin(9600); } void loop() { if (Serial.available()) { int c = Serial.read(); if (c == '0') { myservo.write(0); } else { // Servo write also int number myservo.write(c); } } }