How to convert a PDF from Word with PHPOffice
After I tried some functions from the PhpOffice library, I've finally found an solution.
So with the library at self, you can not load and save it directly from a string to a string. You have to create files currently.
//Set header to show as PDF
header("Content-Type: application/pdf");
header("Content-Disposition: inline; filename=" . $data["name"]);
//Create a temporary file for Word
$temp = tmpfile();
fwrite($temp, $data["data"]); //Write the data in the file
$uri = stream_get_meta_data($temp)["uri"]; //Get the location of the temp file
//Convert the docx file in to an PhpWord Object
$doc = PhpOffice\PhpWord\IOFactory::load($uri);
//Set the PDF Engine Renderer Path. Many engines are supported (TCPDF, DOMPDF, ...).
\PhpOffice\PhpWord\Settings::setPdfRendererPath("path/to/tcpdf");
\PhpOffice\PhpWord\Settings::setPdfRendererName('TCPDF');
//Create a writer, which converts the PhpWord Object into an PDF
$xmlWriter = \PhpOffice\PhpWord\IOFactory::createWriter($doc, 'PDF');
//Create again an temp file for the new generated PDF.
$pdf_temp = tmpfile();
$pdf_uri = stream_get_meta_data($pdf_temp)["uri"];
//Save the PDF to the path
$xmlWriter->save($pdf_uri);
//Now print the file from the temp path.
echo file_get_contents($pdf_uri);
I choose the TCPDF engine, because it was very easy to install. Just simply download the files from the Git Repository or the website and load it in to an folder.
Of course, finally it might be not the best solution, but for me, it is just a preview of an word document.
So this script was inspired also by the following two links:
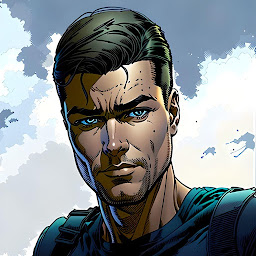
Julian Schmuckli
Developing different apps for different devices.
Updated on June 04, 2022Comments
-
Julian Schmuckli almost 2 years
I don't have any plan how can I convert an Word document, which I have as a string (blob) from my database, to a PDF via the PHPOffice library.
Concept:
- I fetch an existing word document as an string from the database
- Pass the string through an constructor or an function of PHPOffice library.
- Then get via another function the PDF as an string
- And last output the string with
Content-Type: application/pdf
to the user.
Nr. 1 and 4 I already implemented. But I don't know how to accomplish nr. 2 and 3. Can someone help me?
Code:
//DB-Connection, Composer autoload, ... $id = htmlspecialchars(trim($_REQUEST["id"])); $get_data = $con->query("SELECT * FROM word_documents WHERE id='$id'"); //Get the blob $data = $get_data->fetch(); if ($get_data->rowCount() == 1) { header("Content-Type: application/pdf"); header("Content-Disposition: inline; filename=" . $data["name"]); //TODO: Print the PDF as an string } else { echo "File not found"; }
-
King Reload almost 7 yearssome code might help us solve your problem quicker :)
-
Julian Schmuckli almost 7 years@KingReload Thank's for your comment. I uploaded some code of my existing implementation.
-
King Reload almost 7 yearsThanks for your code :) appreciate it