How to convert byte array to any type
Solution 1
Primitive types are easy because they have a defined representation as a byte array. Other objects are not because they may contain things that cannot be persisted, like file handles, references to other objects, etc.
You can try persisting an object to a byte array using BinaryFormatter
:
public byte[] ToByteArray<T>(T obj)
{
if(obj == null)
return null;
BinaryFormatter bf = new BinaryFormatter();
using(MemoryStream ms = new MemoryStream())
{
bf.Serialize(ms, obj);
return ms.ToArray();
}
}
public T FromByteArray<T>(byte[] data)
{
if(data == null)
return default(T);
BinaryFormatter bf = new BinaryFormatter();
using(MemoryStream ms = new MemoryStream(data))
{
object obj = bf.Deserialize(ms);
return (T)obj;
}
}
But not all types are serializable. There's no way to "store" a connection to a database, for example. You can store the information that's used to create the connection (like the connection string) but you can't store the actual connection object.
Solution 2
you can deserialize the byte array to a class object like this in .Net 6.
using System.Text.Json;
using MemoryStream ms = new MemoryStream(bytes);
Examlple ex = JsonSerializer.Deserialize<Example>(ms);
Console.WriteLine(ex.Value);
class Example
{
string Value {get; set; }
}
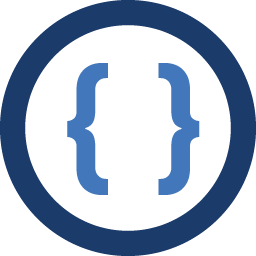
Admin
Updated on October 26, 2021Comments
-
Admin over 2 years
okay guys I'm seeing question from persons asking how to convert byte arrays to
int
,string
,Stream
, etc... and the answers to which are all varying and I have personally not found any satisfactory answers.So here are some types that we want to convert an array of bytes to.
UnityEngine.Font
which can take inttf
data.UnityEngine.Testure2D
which h can take in data from image files like.png
,.jpg
, etc...How would we convert a byte array to a
String
,UnityEngine.Testure2D,UnityEngine.Font
,Bitmap
, etc...The data that populates the byte array must be from a file type whose data can by managed by the type we want to convert the byte array to?
Is this currently possible?
Any help would be appreciated.