How to convert QString to std::string?
Solution 1
One of the things you should remember when converting QString
to std::string
is the fact that QString
is UTF-16 encoded while std::string
... May have any encodings.
So the best would be either:
QString qs;
// Either this if you use UTF-8 anywhere
std::string utf8_text = qs.toUtf8().constData();
// or this if you're on Windows :-)
std::string current_locale_text = qs.toLocal8Bit().constData();
The suggested (accepted) method may work if you specify codec.
See: http://doc.qt.io/qt-5/qstring.html#toLatin1
Solution 2
You can use:
QString qs;
// do things
std::cout << qs.toStdString() << std::endl;
It internally uses QString::toUtf8() function to create std::string, so it's Unicode safe as well. Here's reference documentation for QString
.
Solution 3
If your ultimate aim is to get debugging messages to the console, you can use qDebug().
You can use like,
qDebug()<<string;
which will print the contents to the console.
This way is better than converting it into std::string
just for the sake of debugging messages.
Solution 4
QString qstr;
std::string str = qstr.toStdString();
However, if you're using Qt:
QTextStream out(stdout);
out << qstr;
Solution 5
Best thing to do would be to overload operator<< yourself, so that QString can be passed as a type to any library expecting an output-able type.
std::ostream& operator<<(std::ostream& str, const QString& string) {
return str << string.toStdString();
}
Related videos on Youtube
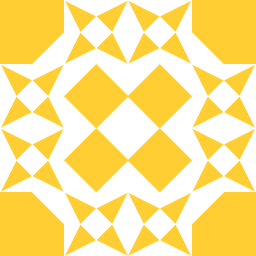
Comments
-
augustin almost 3 years
I am trying to do something like this:
QString string; // do things... std::cout << string << std::endl;
but the code doesn't compile. How to output the content of qstring into the console (e.g. for debugging purposes or other reasons)? How to convert
QString
tostd::string
? -
augustin about 12 yearsI had tried out << qstr first, before asking, but it didn't compile. It works with qstr.toStdString(), though.
-
augustin about 12 yearsWhy the down votes, folks? It's an overkill in my case, but who knows, it might be useful (to me or someone else).
-
chris about 12 yearsI don't think so. You tried std::cout << qstr, not QTextString(stdout) << qstr;
-
Kamil Klimek about 12 yearsqDebug() would be much better, because it supports more Qt types.
-
Vitali about 11 yearsThis isn't safe & is slightly slower than the proper way. You're accessing the data of a QByteArray created on the stack. The destructor for the QByteArray may be called before the constructor of the STL string. The safest way to create a helper function.
static inline std::string toUtf8(const QString& s) { QByteArray sUtf8 = s.toUtf8(); return std::string(sUtf8.constData(), sUtf8.size()); }
-
Artyom about 11 years@Vitali not correct. "The destructor for the QByteArray may be called before the constructor of the STL string" is not correct statement: Quoting the standard: 12.2.3 Temporary objects are destroyed as the last step in evaluating the full-expression (1.9) that (lexically) contains the point where they were created. And the full expression there is
std::string utf8_text = qs.toUtf8().constData();
So your statement is not correct -
Vitali about 11 yearsThat's true - I was thinking about const char *x = qs.ToUtf8().constData(). Still, isn't it easier to just call qs.toStdString()?
-
Notinlist about 11 years@Vitali No. That loses non-latin1 characters. Try this:
QString s = QString::fromUtf8("árvíztűrő tükörfúrógép ÁRVÍZTŰRŐ TÜKÖRFÚRÓGÉP"); std::cout << s.toStdString() << std::endl; std::cout << s.toUtf8().constData() << std::endl;
. The first is incorrect, the second is perfect. You need an utf8 terminal to test this. -
Daniel Saner about 10 yearsFor what it's worth,
.toStdString()
for me always results in an access violation in the pipe operator, irrespective of theQString
's contents (non-latin1 or not). This is on Qt 4.8.3/MSVC++ 10/Win 7. -
Emile Cormier over 9 yearsAs of Qt 5.0,
QString::toStdString()
now usesQString::toUtf8()
to perform the conversion, so the Unicode properties of the string will not be lost (qt-project.org/doc/qt-5.0/qtcore/qstring.html#toStdString). -
Senči over 9 yearsthis works even with a Qt-Build with
-no-stl
-Option set. some more info -
thuga about 8 yearsAnd if you want to check the source code for
QString::toStdString
, here it is. -
Tarod over 7 years@Artyom The answer should be edited because
QByteArray QString::toAscii() const
is obsolete -
Yousuf Azad over 6 yearsHow can i test to see if it indeed loses the Unicode property? Will using Unicode characters(e.g. say from a language other than English) will do?
-
Anantha Raju C almost 6 yearsplease add some details as to what the mistake was and why your answer works
-
eyllanesc about 4 yearsThe question is very clear: convert QString to std::string, not to print it.
-
Den-Jason over 3 yearsI like this because Qt have a habit of changing the way their strings work - and this puts the conversion in one place.
-
Laszlo about 3 years@notinlist Well, both methods result in the same giberish: "�rv�zt?r? t�k�rf�r�g�p �RV�ZT?R? T�K�RF�R�G�P". They are the same at least.
-
M.M almost 3 years@eyllanesc the question text says "How to output the content of qstring into the console?" , it seems OP assumes converting to std::string is the only way. It's really two questions being asked at once .
-
eyllanesc almost 3 years@M.M The question seems unclear since the question in the title says How to convert QString to std::string?, Maybe it's an XY problem.
-
IceBerg0 over 1 yearstackoverflow.com/a/4214397/8533873 should be the accepted answer.