how to count the seconds in java?
Solution 1
You can use either long
values with milliseconds since epoch, or java.util.Date
objects (which internally uses long
values with milliseconds since epoch, but are easier to display/debug).
// Using millis
class MyObj {
private final long createdMillis = System.currentTimeMillis();
public int getAgeInSeconds() {
long nowMillis = System.currentTimeMillis();
return (int)((nowMillis - this.createdMillis) / 1000);
}
}
// Using Date
class MyObj {
private final Date createdDate = new java.util.Date();
public int getAgeInSeconds() {
java.util.Date now = new java.util.Date();
return (int)((now.getTime() - this.createdDate.getTime()) / 1000);
}
}
Solution 2
When you create your object call.
Date startDate = new Date();
After you are done call;
Date endDate = new Date();
The number of seconds elapsed is:
int numSeconds = (int)((endDate.getTime() - startDate.getTime()) / 1000);
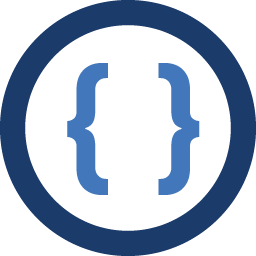
Admin
Updated on July 15, 2022Comments
-
Admin almost 2 years
I'm trying to understand how I could go about keeping track of the seconds that an object has been created for.
The program I'm working on with simulates a grocery store.
Some of the foods posses the trait to spoil after a set amount of time and this is all done in a subclass of an
item
class calledgroceryItem
. The seconds do not need to be printed but are kept track of using acurrentTime
field and I don't quite understand how to count the seconds exactly.I was looking at using the Java.util.Timer or the Java.util.Date library maybe but I don't fully understand how to use them for my issue.
I don't really have a very good understanding of java but any help would be appreciated.