How to create a custom Dialog in flutter
For it, we create a custom dialog
1. Custom Dialog Content class
class CustomDialog extends StatelessWidget {
dialogContent(BuildContext context) {
return Container(
decoration: new BoxDecoration(
color: Colors.white,
shape: BoxShape.rectangle,
borderRadius: BorderRadius.circular(10),
boxShadow: [
BoxShadow(
color: Colors.black26,
blurRadius: 10.0,
offset: const Offset(0.0, 10.0),
),
],
),
child: Column(
mainAxisSize: MainAxisSize.min, // To make the card compact
children: <Widget>[
Image.asset('assets/images/image.jpg', height: 100),
Text(
"Text 1",
style: TextStyle(
fontSize: 24.0,
fontWeight: FontWeight.w700,
),
),
SizedBox(height: 16.0),
Text(
"Text 1",
style: TextStyle(
fontSize: 24.0,
fontWeight: FontWeight.w700,
),
),
SizedBox(height: 24.0),
Align(
alignment: Alignment.bottomCenter,
child: Icon(Icons.cancel),
),
],
),
);
}
@override
Widget build(BuildContext context) {
return Dialog(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10),
),
elevation: 0.0,
backgroundColor: Colors.transparent,
child: dialogContent(context),
);
}
}
2. Call Custom Dialog on Click:
RaisedButton(
color: Colors.redAccent,
textColor: Colors.white,
onPressed: () {
showDialog(
context: context,
builder: (BuildContext context) {
return CustomDialog();
});
;
},
child: Text("PressMe"),
),
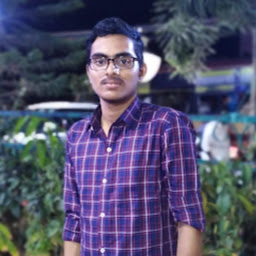
S M Vaidhyanathan
I am a Computer Science and Engineering graduate from Rajalakshmi Engineering College. I am a passionate Software Developer who always loves to learn new skills and apply them in building amazing products. I love to delve deep into any domain that interests me and I make sure that I learn things by understanding them very well. I love to ask a ton of questions in the process of knowing about or learning something.
Updated on December 22, 2022Comments
-
S M Vaidhyanathan over 1 year
I want to create a custom dialog as shown below. I am able to create a normal dialog with two buttons(the positive and negative buttons). But I searched a lot about creating custom dialog like the one shown below but in vain.
showAlertDialog(BuildContext context) { // set up the buttons Widget cancelButton = FlatButton( child: Text("Cancel"), onPressed: () {}, ); Widget continueButton = FlatButton( child: Text("Continue"), onPressed: () {}, ); // set up the AlertDialog AlertDialog alert = AlertDialog( title: Text("Action"), content: Text("Would you like to continue learning how to use Flutter alerts?"), actions: [ cancelButton, continueButton, ], ); // show the dialog showDialog( context: context, builder: (BuildContext context) { return alert; }, ); }
Now I want to have these buttons and the image as the children of the dialog and the icon button 'X' in the bottom to close the dialog. Any help is appreciated. I am a complete beginner in flutter.