How to delete an item from UICollectionView with indexpath.row
44,287
Solution 1
-(void)remove:(int)i {
[self.collectionObj performBatchUpdates:^{
[array removeObjectAtIndex:i];
NSIndexPath *indexPath =[NSIndexPath indexPathForRow:i inSection:0];
[self.collectionObj deleteItemsAtIndexPaths:[NSArray arrayWithObject:indexPath]];
} completion:^(BOOL finished) {
}];
}
Try this. It may work for you.
Solution 2
Swift 3 solution:
func remove(_ i: Int) {
myObjectsList.remove(at: i)
let indexPath = IndexPath(row: i, section: 0)
self.collectionView.performBatchUpdates({
self.collectionView.deleteItems(at: [indexPath])
}) { (finished) in
self.collectionView.reloadItems(at: self.collectionView.indexPathsForVisibleItems)
}
}
and example delete call:
self.remove(indexPath.row)
Solution 3
swift 3:
func remove(index: Int) {
myObjectList.remove(at: index)
let indexPath = IndexPath(row: index, section: 0)
collectionView.performBatchUpdates({
self.collectionView.deleteItems(at: [indexPath])
}, completion: {
(finished: Bool) in
self.collectionView.reloadItems(at: self.collectionView.indexPathsForVisibleItems)
})
}
Solution 4
[array removeObjectAtIndex:[indexPath row]];
[collection reloadData]; // Collection is UICollectionView
Try this.
Solution 5
[array removeObjectAtIndex:[indexPath row]];
[self.collectionView deleteItemsAtIndexPaths:@[indexPath]];
Normally it's ok... You can see this post, it deals with same subject.
Related videos on Youtube
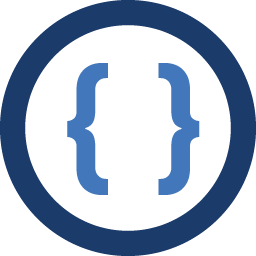
Author by
Admin
Updated on June 24, 2020Comments
-
Admin about 4 years
I have a collection view,and I tried to delete a cell from collection view on didSelect method.I succeeded in that using the following method
[colleVIew deleteItemsAtIndexPaths:[NSArray arrayWithObject:indexPath]];
But now I need to delete the item on button click from CollectionView Cell.Here I get only the indexpath.row. From this I am not able to delete the Item. I tried like this.
-(void)remove:(int)i { NSLog(@"index path%d",i); [array removeObjectAtIndex:i]; NSIndexPath *indexPath =[NSIndexPath indexPathForRow:i inSection:0]; [colleVIew deleteItemsAtIndexPaths:[NSArray arrayWithObject:indexPath]]; [colleVIew reloadData]; }
But it needs to reload the CollectionView.So the animation of cell arrangement after deletion is not there. Please suggest an idea..thanks in advance
-
Admin about 11 yearsbut I am not getting the indexpath I assigns i = indexpath.row;
-
Divyam shukla about 11 years@NithinMK you have to assign the indexpath for getting the reference of the cell.
-
Jagat Dave over 8 yearsThanks...It is help me and save my time.
-
Lance Samaria over 3 yearsThe key to this problem that this answer addresses is both the item to remove from the array and the item to delete from the collectionView both occur inside
performBatchUpdates