How to display images from a external storage path in flutter?
Solution 1
Provide your storage path and you can display image
Image.file(File(imagePath);
Solution 2
I've tried following a similar approach as yours for fetching files by using path_provider
. However, the plugin is only able to fetch the app's directory, even when using getExternalStorageDirectory()
. This behavior is also pointed out in this Stack Overflow post.
Instead, I've used ext_storage
to access the device's storage.
This sample app demonstrates fetching files on your device's /Download folder using ext_storage
, handling permissions using permission_handler
, file extension check, and displaying images from path on a GridView.
import 'dart:io';
import 'package:flutter/material.dart';
import 'package:ext_storage/ext_storage.dart';
import 'package:permission_handler/permission_handler.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
Future _futureGetPath;
List<dynamic> listImagePath = List<dynamic>();
var _permissionStatus;
@override
void initState() {
super.initState();
_listenForPermissionStatus();
// Declaring Future object inside initState() method
// prevents multiple calls inside stateful widget
_futureGetPath = _getPath();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Expanded(
flex: 1,
child: FutureBuilder(
future: _futureGetPath,
builder: (BuildContext context, AsyncSnapshot snapshot) {
if (snapshot.hasData) {
var dir = Directory(snapshot.data);
print('permission status: $_permissionStatus');
if (_permissionStatus) _fetchFiles(dir);
return Text(snapshot.data);
} else {
return Text("Loading");
}
},
),
),
Expanded(
flex: 19,
child: GridView.count(
primary: false,
padding: const EdgeInsets.all(20),
crossAxisSpacing: 10,
mainAxisSpacing: 10,
crossAxisCount: 3,
children: _getListImg(listImagePath),
),
)
],
),
);
}
// Check for storage permission
void _listenForPermissionStatus() async {
final status = await Permission.storage.request().isGranted;
// setState() triggers build again
setState(() => _permissionStatus = status);
}
// Get storage path
// https://pub.dev/documentation/ext_storage/latest/
Future<String> _getPath() {
return ExtStorage.getExternalStoragePublicDirectory(
ExtStorage.DIRECTORY_DOWNLOADS);
}
_fetchFiles(Directory dir) {
List<dynamic> listImage = List<dynamic>();
dir.list().forEach((element) {
RegExp regExp =
new RegExp("\.(gif|jpe?g|tiff?|png|webp|bmp)", caseSensitive: false);
// Only add in List if path is an image
if (regExp.hasMatch('$element')) listImage.add(element);
setState(() {
listImagePath = listImage;
});
});
}
List<Widget> _getListImg(List<dynamic> listImagePath) {
List<Widget> listImages = List<Widget>();
for (var imagePath in listImagePath) {
listImages.add(
Container(
padding: const EdgeInsets.all(8),
child: Image.file(imagePath, fit: BoxFit.cover),
),
);
}
return listImages;
}
}
Note that this only covers the basics of displaying images on a GridView. To scale, I suggest following this guide on performance best practices.
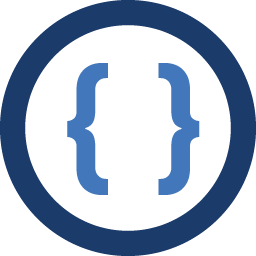
Admin
Updated on December 08, 2022Comments
-
Admin over 1 year
Im basically new to flutter. I want to display Images from specific path like "Storage/WhatsApp/Media/". I want to show all the images in grid view. How can i acheive this in flutter. I have seen many examples but everyone is using assets folder. This is the code for getting the path. How to display them in grid view?
Future<String> get localpath async { final dir=await getExternalStorageDirectory(); return dir.path; } Future<File> get localfile async{ final path=await localpath; return File('$path/WhatsApp/Media/WhatsApp Images'); } Future<String> readData() async{ try{ final file=await localfile; String image_path=await file.readAsString(); return image_path; } catch(e) {return e.toString();} }
Now since i got the path how to display images in gridview? Should i use gridview.builder?
-
Oswin Noetzelmann over 5 years
-
Admin over 5 yearsYeah I got it. But suppose the directory has 10 images like 'WA001.JPG,WA004.JPG'. Should I create an array list and send it to FileImage?
-
Oswin Noetzelmann over 5 yearsNo, the Gridview.builder uses the list to build 10 FileImages inside the itembuilder property - compare the example on the blog I linked.
-