How to find current location of the user using map_view in flutter?
1,859
First you can hard code the position you want to show for default:
MapView mapView = new MapView();
mapView.show(
new MapOptions(
title: 'map title',
showUserLocation: true,
initialCameraPosition: CameraPosition(new Location(35.7050274, 51.364935),11.92)
)
);
then bind a function to map view so when gps find user location camera position get updated:
mapView.onLocationUpdated.lastWhere((position)=>this.onUserLocationUpdated(position,mapView));
the method name lastWhere
is called once on user location update if you want to track user movement you can use listen
instead.
after that you just need to define a function that change camera position to user current position :
onUserLocationUpdated(position,MapView mapview)async {
double zoomLevel = await mapview.zoomLevel;
mapview.setCameraPosition(position.latitude, position.longitude, zoomLevel);
}
in above code we get user zoom level so every movement dont change current zoom level of user .
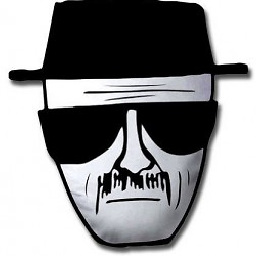
Author by
Keshav Aditya R.P
Updated on December 04, 2022Comments
-
Keshav Aditya R.P 8 months
I would like to set initialCameraPosition to point to the user's current position rather than hard code a longitude and latitude.
showMap() { mapView.show( new MapOptions( mapViewType: MapViewType.normal, showUserLocation: true, initialCameraPosition: new CameraPosition( new Location(23.2599, 77.4126), 10.0), title: "Parking Spaces"), toolbarActions: [new ToolbarAction("Close", 1)] );