How to find Sidekiq is running or not
Solution 1
A little trick:
ps aux | grep '[s]idekiq'
Hope it works
Solution 2
Ideally you can do this directly from ruby itself. Put this in some rake task or standalone script (don't forget to specify Sidekiq connection details)
ps = Sidekiq::ProcessSet.new
ps.size # => 2
ps.each do |process|
p process['busy'] # => 3
p process['hostname'] # => 'myhost.local'
p process['pid'] # => 16131
end
ps.each(&:quiet!) # equivalent to the USR1 signal
ps.each(&:stop!) # equivalent to the TERM signal
From https://github.com/mperham/sidekiq/wiki/API#processes
Solution 3
See this question for how to filter ps output using grep, while eliminating the grep command from the the output.
Solution 4
I see, try this out:
module Process
class << self
def is_running?(pid)
begin
Process.kill(0, pid)
true
rescue Errno::ESRCH
false
end
end
end
end
1.9.3p392 :001 > puts `ps aux | grep -i [s]idekiq`
It'll return you pid like: 12247, and you can check if it's running:
Process.is_running?(12247) // true | false
Solution 5
You can add the following lines to your config/routes.rb
require 'sidekiq/web'
mount Sidekiq::Web => '/sidekiq'
Start your server, open a browser and check your processess and your jobs
You can find it from Sidekiq Wiki
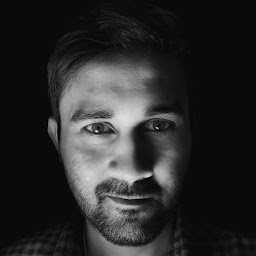
AnkitG
I am working with realtor.com, A Ruby and Ruby on Rails enthusiast and a Rails Contributor, Agile and a scrum practitioner and a Photographer. Creator of followg gems: Carrierwave Encrypter Decrypter Days Picker Saturday HighStocks rails Piecon Rails Font Awesome icons list
Updated on January 18, 2020Comments
-
AnkitG over 4 years
In one of my project i am using Sidekiq
Is there any inbuilt Sidekiq console method/method that helps me to find whether sidekiq is running or not.
My requirement is kind of a pre check condition where if Sidekiq is not running i will raise a error.
I tried using the grep like
'ps -ef | grep sidekiq'
but it's not solving my purpose.
The method i am looking for should be something like:
Sidekiq.is_running?
Thanks in advance.
I also Tried
Sidekiq not running
1.9.3p392 :021 > system 'ps aux | grep sidekiq' ankitgupta 6683 0.0 0.0 2432768 600 s001 R+ 11:47AM 0:00.00 grep sidekiq ankitgupta 6681 0.0 0.0 2433432 916 s001 S+ 11:47AM 0:00.01 sh -c ps aux | grep sidekiq => true
Sidekiq is running
1.9.3p392 :022 > system 'ps aux | grep sidekiq' ankitgupta 6725 0.0 0.0 2432768 600 s001 S+ 11:57AM 0:00.00 grep sidekiq ankitgupta 6723 0.0 0.0 2433432 916 s001 S+ 11:57AM 0:00.00 sh -c ps aux | grep sidekiq ankitgupta 6707 0.0 1.3 3207416 111608 s002 S+ 11:56AM 0:07.46 sidekiq 2.11.2 project_name [0 of 25 busy] => true
It is always returning true.. I want to catch the process when it runs