How to find the index of an element in an array in Java?
Solution 1
In this case, you could create e new String from your array of chars and then do an indeoxOf("e") on that String:
System.out.println(new String(list).indexOf("e"));
But in other cases of primitive data types, you'll have to iterate over it.
Solution 2
Alternatively, you can use Commons Lang ArrayUtils class:
int[] arr = new int{3, 5, 1, 4, 2};
int indexOfTwo = ArrayUtils.indexOf(arr, 2);
There are overloaded variants of indexOf()
method for different array types.
Solution 3
For primitive arrays
Starting with Java 8, the general purpose solution for a primitive array arr
, and a value to search val
, is:
public static int indexOf(char[] arr, char val) {
return IntStream.range(0, arr.length).filter(i -> arr[i] == val).findFirst().orElse(-1);
}
This code creates a stream over the indexes of the array with IntStream.range
, filters the indexes to keep only those where the array's element at that index is equal to the value searched and finally keeps the first one matched with findFirst
. findFirst
returns an OptionalInt
, as it is possible that no matching indexes were found. So we invoke orElse(-1)
to either return the value found or -1
if none were found.
Overloads can be added for int[]
, long[]
, etc. The body of the method will remain the same.
For Object arrays
For object arrays, like String[]
, we could use the same idea and have the filtering step using the equals
method, or Objects.equals
to consider two null
elements equal, instead of ==
.
But we can do it in a simpler manner with:
public static <T> int indexOf(T[] arr, T val) {
return Arrays.asList(arr).indexOf(val);
}
This creates a list wrapper for the input array using Arrays.asList
and searches the index of the element with indexOf
.
This solution does not work for primitive arrays, as shown here: a primitive array like int[]
is not an Object[]
but an Object
; as such, invoking asList
on it creates a list of a single element, which is the given array, not a list of the elements of the array.
Solution 4
That's not even valid syntax. And you're trying to compare to a string. For arrays you would have to walk the array yourself:
public class T {
public static void main( String args[] ) {
char[] list = {'m', 'e', 'y'};
int index = -1;
for (int i = 0; (i < list.length) && (index == -1); i++) {
if (list[i] == 'e') {
index = i;
}
}
System.out.println(index);
}
}
If you are using a collection, such as ArrayList<Character>
you can also use the indexOf()
method:
ArrayList<Character> list = new ArrayList<Character>();
list.add('m');
list.add('e');
list.add('y');
System.out.println(list.indexOf('e'));
There is also the Arrays
class which shortens above code:
List list = Arrays.asList(new Character[] { 'm', 'e', 'y' });
System.out.println(list.indexOf('e'));
Solution 5
I believe the only sanest way to do this is to manually iterate through the array.
for (int i = 0; i < list.length; i++) {
if (list[i] == 'e') {
System.out.println(i);
break;
}
}
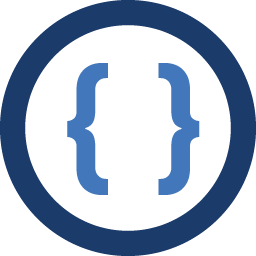
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
I am looking to find the index of a given element, knowing its contents, in Java.
I tried the following example, which does not work:
class masi { public static void main( String[] args ) { char[] list = {'m', 'e', 'y'}; // should print 1 System.out.println(list[] == "e"); } }
Can anyone please explain what is wrong with this and what I need to do to fix it?
-
Rich over 14 yearsI very much doubt there is a
list
type you can build an array from. If there is, naming conventions are gravely violated. -
skaffman over 14 years
List
defineas atoArray
method -
Michal aka Miki over 14 yearsI need to have alphabets in the array like: a -> 1, b -> 2,..., z -> 26. - I am coding just for fun, not for homework assignment.
-
Michal aka Miki over 14 yearsYour solution suggests me that I need to have 26 hashMap commands. Is there any way of combining the hashmaps commands?
-
Rich over 14 yearsThat was a reply to aperkins who apparently gave me a downvote for my statement that
list[] == "e"
was invalid syntax. For the[]
to work,list
has to be a type, not a variable. That's what I meant with "list
type to build an array from". Sorry for the misunderstanding. -
Michal aka Miki over 14 yearsThe order matters in my case. My example is more simple than the problem which I have currently in hand. I have 26 letters actually in the list from a, b, ... to z.
-
Bill K over 14 yearsHey, if you just want to know if a is 1, b is 2, just use "charValue-'a'+1"! I thought you wanted to actually have different values, or store things in buckets.
-
Bogdan over 9 yearsThis is the best way to do it. If you don't have apache in your project, add it. Another way is to use java.util.Arrays.binarySearch but it needs a sorted array, so not always useful.
-
4castle over 7 yearsDon't convert to an
ArrayList
, and don't calllist.clear()
. Those are iterating the array 2 extra times. -
Gili Ariel almost 4 yearsCan you also write here the full implementation to add to the project?
-
Nico Haase over 3 yearsPlease add some explanation to your answer such that others can learn from it
-
Ali Ali over 3 yearsYour code will count how many times the 'e' char found in the string, not giving the index.
-
Arun Joseph over 2 yearsBe careful, this will only work of char Array as the length of each character will be 1.