How to find the indices of all elements matching from one list to another in Dart/Flutter
Solution 1
Try this:
main() {
var firstList = ["Travel", "Shopping", "Transport"];
var secondList = [
"Travel",
"Shopping",
"Shopping",
"Travel",
"Transport",
"Transport",
"Travel",
"Travel"
];
var thirdList = [];
for (var i = 0; i < secondList.length; i++) {
if (secondList[i] == "Travel") {
thirdList.add(i);
}
}
print(thirdList);
}
EDIT: For it to work for every item in firstList
Try this:
main() {
var firstList = ["Travel", "Shopping", "Transport"];
var secondList = [
"Travel",
"Shopping",
"Shopping",
"Travel",
"Transport",
"Transport",
"Travel",
"Travel"
];
var thirdList = [];
for (var i = 0; i < firstList.length; i++) {
var sublist = [];
for (var j = 0; j < secondList.length; j++) {
if (secondList[j] == firstList[i]) {
sublist.add(j);
}
}
thirdList.add(sublist);
}
print(thirdList); // [[0, 3, 6, 7], [1, 2], [4, 5]]
}
Solution 2
List firstList = ["Travel", "Shopping", "Transport"];
List secondList = ["Travel", "Shopping", "Shopping", "Travel", "Transport", "Transport", "Travel", "Travel"];
List indexList = [];
getElement() {
for (int i = 0; i <= secondList.length; i++) {
if (secondList[i] == "Travel") {
indexList.add(i);
}
}
print(indexList);
}
Solution 3
void matchItem()
{
var firstList = ["Travel", "Shopping", "Transport"];
var secondList = ["Travel", "Shopping", "Shopping", "Travel", "Transport", "Transport", "Travel", "Travel"];
var foundItemPositions = [];
for(int i=0;i<firstList.length;i++)
{
if(secondList.contains(firstList[i]))
{
for(int j=0;j<secondList.length;j++)
{
if(firstList[i] == secondList[j])
{
foundItemPositions.add(j);
}
}
}
}
print(foundItemPositions);
}
Solution 4
we can achieve this with a single loop.
Try this
for (int j = 0; j < secondList.length; j++) {
if (secondList[j] == 'Travel') indexList.add(j);
}
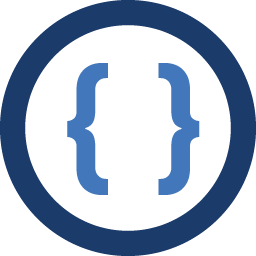
Admin
Updated on January 03, 2023Comments
-
Admin over 1 year
Suppose, I have a list:
firstList = ["Travel", "Shopping", "Transport"];
another list:
secondList = ["Travel", "Shopping", "Shopping", "Travel", "Transport", "Transport", "Travel", "Travel"]
I need to find the index of all the elements from the secondList that contains:
"Travel"
Here in
secondList
"Travel"
is present in index 0, 3, 6, and 7Now, I have a 3rd List containing the index of the element, here
"Travel"
.indexList = [0, 3, 6, 7]
// Since index 0, 3, 6, and 7 only contains the element"Travel"
.Below is my program:
for (int i = 0; i < firstList.length; i++) { for (int j = 0; j < secondList.length; j++) { indexList.add(secondList.indexOf(firstList[i])); } }
This is not working as I am getting output like this:
[0, 0, 0, 0, 0, 0, 0, 0, 0]
Seems like it stuck on the first index itself.
The "Travel", here is an example, it should be matching dynamically such, firstList[i] or any other element not just "Travel" as hardcoded. Such as if I have selected firstList[i] then find the index of the same element in the secondList[].
Please help me to identify the cause. I am new to programming.
-
kl23 over 2 yearsThe "Travel", here is an example, it should be matching dynamically such as, firstList[i] or any other element not just "Travel" as hardcoded. Such as if I have selected firstList[i] then find the index of the same element in the secondList[].
-
Ravi Limbani over 2 yearsyou can get any from firstList but you need to store alls result in indexList. You need to use if condition like if(firstList[i] == secondList[i]){
-
Josteve over 2 yearsCheck this out: stackoverflow.com/a/70893478/11452511
-
-
Josteve over 2 yearsFrom effective dart, it is recommended to not use
forEach
in this fashion. Use the normalfor loop
instead; Check this: dart.dev/guides/language/effective-dart/… -
Farman Ali Khan over 2 yearsThanks @Josteve , it's updated now.