How to find the numbers in the thousands, hundreds, tens, and ones place in PYTHON for an input number? For example: 256 has 6 ones, 5 tens, etc
42,897
Solution 1
You might want to try something like following:
def get_pos_nums(num):
pos_nums = []
while num != 0:
pos_nums.append(num % 10)
num = num // 10
return pos_nums
And call this method as following.
>>> get_pos_nums(9876)
[6, 7, 8, 9]
The 0th
index will contain the units, 1st
index will contain tens, 2nd
index will contain hundreds and so on...
This function will fail with negative numbers. I leave the handling of negative numbers for you to figure out as an exercise.
Solution 2
Like this?
a = str(input('Please give me a number: '))
for i in a[::-1]:
print(i)
Demo:
Please give me a number: 1324
4
2
3
1
So the first number is ones, next is tens, etc.
Solution 3
You could try splitting the number using this function:
def get_place_values(n):
return [int(value) * 10**place for place, value in enumerate(str(n)[::-1])]
For example:
get_place_values(342)
>>> [2, 40, 300]
Next, you could write a helper function:
def get_place_val_to_word(n):
n_str = str(n)
num_to_word = {
"0": "ones",
"1": "tens",
"2": "hundreds",
"3": "thousands"
}
return f"{n_str[0]} {num_to_word[str(n_str.count('0'))]}"
Then you can combine the two like so:
def print_place_values(n):
for value in get_place_values(n):
print(get_place_val_to_word(value))
For example:
num = int(input("Please give me a number: "))
# User enters 342
print_place_values(num)
>>> 2 ones
4 tens
3 hundreds
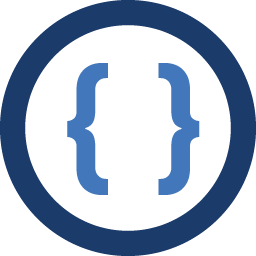
Author by
Admin
Updated on July 21, 2022Comments
-
Admin almost 2 years
num = int(input("Please give me a number: ")) print(num) thou = int((num // 1000)) print(thou) hun = int((num // 100)) print(hun) ten =int((num // 10)) print(ten) one = int((num // 1)) print(one)
I tried this but it does not work and I'm stuck.
-
hectorcanto about 3 yearsA code-styled, function-based program might be better:
python def tens_of_number(number: int): return number % 100 / 10 num = 1234 print("tens of ", num, ":", tens_of_number(num))
-
Santosh Kumar about 2 yearsThis is a good hack. But interviewers don't want this.