How to fix "One or more validation errors were detected during model generation"-error
Solution 1
By convention EF uses either the field Id
or [type name]Id
as a Primary Key. See here: http://msdn.microsoft.com/en-us/library/system.data.entity.modelconfiguration.conventions.idkeydiscoveryconvention%28v=vs.103%29.aspx
The fact that your type is named shop_Products
but you have the key set to ProductID
(assumption) means it cant find one by convention. So you can either change the field name or type name or add the data annotation [Key]
above ProductID
like so:
[Key]
public int ProductID { get; set; }
Solution 2
Add this namespace ref System.ComponentModel.DataAnnotations
to your model and then add the [Key]
annotation above your id property
Solution 3
namespace: using System.ComponentModel.DataAnnotations.Schema;
[Key] public int ProductID { get; set; }
Solution 4
you can also just rename productID to id
public int id {get; set;}
Solution 5
Here is my code for this type of problem in asp.net mvc
before
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace glostars.Models
{
public class WeeklyTagged
{
public int TaggedId { get; set; }
}
}
after
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations; //added this line
using System.Linq;
using System.Web;
namespace glostars.Models
{
public class WeeklyTagged
{
[Key] //and this line
public int TaggedId { get; set; }
}
}
And now it works. Thank you
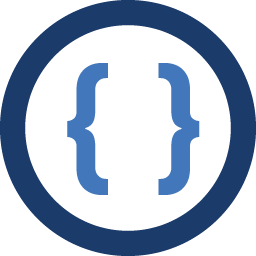
Admin
Updated on December 16, 2020Comments
-
Admin over 3 years
One or more validation errors were detected during model generation:
SportsStore.Domain.Concrete.shop_Products: : EntityType 'shop_Products' has no key defined. Define the key for this EntityType.
Products: EntityType: EntitySet 'Products' is based on type 'shop_Products' that has no keys defined.
public ViewResult Index() { ProductsListViewModel viewModel = new ProductsListViewModel { Products = repository.Products .Where(p => p.CategoryId == 100) .OrderByDescending(p=>p.ProductID) .Take(5) }; return View(viewModel); } @foreach (var p in Model.Products) { <a href="">@p.ProductName</a> } public class shop_Products { public int ProductID { get; set; } public string ProductName { get; set; } public int CategoryId { get; set; } public Nullable<int> CategoryPropertyId { get; set; } public string PropertyValue { get; set; } public Nullable<int> ProductBrandId { get; set; } public Nullable<decimal> MarketPrice { get; set; } public decimal Price { get; set; } public Nullable<decimal> UserPrice { get; set; } public string TitleKeyword { get; set; } public string MetaKeyword { get; set; } public string MetaDescription { get; set; } public string PhotoName { get; set; } public string PhotoPath { get; set; } public string smallPhotos { get; set; } public string BigPhotos { get; set; } public string URL { get; set; } public double Discount { get; set; } public int Inventory { get; set; } public string ShortDesc { get; set; } public bool IsAccessories { get; set; } public bool IsGroup { get; set; } public bool IsTopService { get; set; } public string Accessorices { get; set; } public string PeopleGroup { get; set; } public string TopService { get; set; } public string Contents { get; set; } public string Parameter { get; set; } public string PackingList { get; set; } public string Service { get; set; } public string Professional { get; set; } public bool IsParameter { get; set; } public bool IsPackingList { get; set; } public bool IsService { get; set; } public bool IsProfessional { get; set; } public Nullable<bool> IsEnable { get; set; } public Nullable<bool> IsCommend { get; set; } public Nullable<bool> IsTop { get; set; } public Nullable<bool> IsBest { get; set; } public string ProductBrandType { get; set; } public string Manufacturer { get; set; } public string Makein { get; set; } public string weight { get; set; } public System.DateTime InputTime { get; set; } public Nullable<int> Sort { get; set; } public Nullable<int> SeeCount { get; set; } }
I made a few of these,and works well.But these is wrong.Can anybody help me?
-
Gert Arnold over 5 yearsThree of four existing answers already state this. What's the added value of your answer?
-
MD JULHAS HOSSAIN over 5 yearsThere are so many beginner developer like me . I think if anyone answered like me its more helpful for them. That's why I answered like a beginner way.
-
Gert Arnold over 5 yearsWhich beginner won't understand this answer?
-
MD JULHAS HOSSAIN over 5 yearsBest example that is me :D