how to generate circle using javascript
Solution 1
You need to use the following liabrary:-
Put the code in your head tag.
<script type="text/JavaScript" src="jsDraw2D.js"></script>
It is available to download from here
Copy and paste the following code where you want your circle to appear...
<script type="text/JavaScript">
//Create jsGraphics object
var gr = new jsGraphics(document.getElementById("canvas"));
//Create jsColor object
var col = new jsColor("red");
//Create jsPen object
var pen = new jsPen(col,1);
//Draw a Line between 2 points
var pt1 = new jsPoint(20,30);
var pt2 = new jsPoint(120,230);
gr.drawLine(pen,pt1,pt2);
//Draw filled circle with pt1 as center point and radius 30.
gr.fillCircle(col,pt1,30);
//You can also code with inline object instantiation like below
gr.drawLine(pen,new jsPoint(40,10),new jsPoint(70,150));
</script>
You can check the documentation for this from here
Solution 2
Here's a simple way to do a circle for modern browsers:
<div style='border: 3px solid red; border-radius: 50px; width: 100px; height: 100px;'>
</div>
Demo.
edit — It works better with the "box-sizing" ("-moz-box-sizing" for Firefox) set to "border-box".
<style>
div.circle {
border: 3px solid red;
border-radius: 50%;
width: 100px; height: 100px;
-moz-box-sizing: border-box;
box-sizing: border-box;
}
</style>
<div class=circle>
</div>
Solution 3
Using HTML5 Canvas and AJAX, you can do the following:
window.onload = function(){
var canvas = document.getElementById("myCanvas");
var context = canvas.getContext("2d");
var centerX = canvas.width / 2;
var centerY = canvas.height / 2;
var radius = 10;
context.beginPath();
context.arc(centerX, centerY, radius, 0, 2 * Math.PI, false);
context.fillStyle = "black";
context.fill();
context.lineWidth = 1;
context.strokeStyle = "black";
context.stroke();
};
the big line being the:
context.arc(centerX, centerY, radius, 0, 2 * Math.PI, false);
For more go see: HTML5 Canvas Tutorials
Solution 4
You can use some javascript library for the same. Like here is a third party js library which may serve your purpose
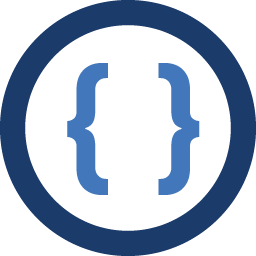
Admin
Updated on September 22, 2020Comments
-
Admin over 3 years
How can i create a Circle in Html using javascript. Does it can be done using Javascript or not? I have done creating rectangles and squares but don't know how to accomplish a circle.
-
JamesUsedHarden over 10 yearsThis draws a circle, but what does it have to do with AJAX?
-
Bob almost 9 yearsthere is no AJAX here (and none needed)
-
thatOneGuy about 8 yearsI don't see the point in downloading extra libraries when this can be done easily in css as shown below or just as easy using canvas
-
thatOneGuy over 7 years@MahdiJazini and what suggestion is that ?