how to get data from cloud firestore where user.uid equal to document id in flutter?
Solution 1
If the document id in your firestore is equal to the userid
in the Firebase authentication console, then you need to retrieve the uid
first and pass it as an argument to the method document()
:
getData() async{
String userId = (await FirebaseAuth.instance.currentUser()).uid;
return Firestore.instance.collection('users').document(userId);
}
Solution 2
Your query is fetching all of the documents in the "userData" collection, then picking out the first document from that entire set. This will be the same set of documents for all users that have read access to that collection. I don't see why you would expect a different result for different users. Perhaps you meant to access a single document for a user given their user ID, instead of all of the documents. If that's the case, you should request that document by its ID with Firestore.instance.collection('userData').document(uid)' where
uid` is the ID of the currently signed in user.
Also, your code is querying a collection called "userData", but your screenshot shows a collection called "users", so that is confusing.
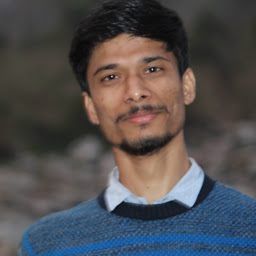
Sandeep Sharma
Updated on December 17, 2022Comments
-
Sandeep Sharma over 1 year
I am Having this profile screen which shows users info. after user authenticated I am storing data in cloud firestore with document id is equal to user-id. Now, I want to retrieve data from cloud firestore with having current userId is equal to document id.
For now i have this :
class UserManagement { getData() async{ String userId = (await FirebaseAuth.instance.currentUser()).uid; print(userId); return Firestore.instance.collection('users').document(userId); }
but this is not working properlywhen i log out and after re-login with different user it showing me same data.
UserManagement().getData().then((results) { setState(() { userFlag = true; users = results; }); });
Now, how get other fields like name,email,course,phonenumber..etc and all values all storing into user.right?