Not able to fetch particular user document by user id in flutter app
1,102
When using userID
is doesn't work because currentUser()
is asynchronous and the StreamBuilder
is being called even before getting the userId
therefore try the following:
Stream<DocumentSnapshot> getData()async*{
FirebaseUser user = await FirebaseAuth.instance.currentUser();
yield* Firestore.instance.collection('users').document(user.uid).snapshots();
}
Create a method that returns a Stream
and then inside the StreamBuilder
do the following:
children: <Widget>[
StreamBuilder(
stream: getData(),
builder: (context,snapshot){
if (!snapshot.hasData) return const Text("Loading...");
else if(snapshot.hasData){
return Container(
child: Column(
children: <Widget>[
Text(snapshot.data["name"]),
Text(snapshot.data["email"]),
Text(snapshot.data["phone"].toString()),
],
),
);
},
return CircularProgressIndicator();
},
)
],
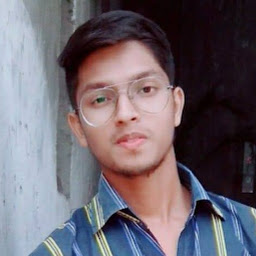
Author by
Santosh Kp
Updated on December 22, 2022Comments
-
Santosh Kp over 1 year
I want to retrieve a particular user document by its id from the collection users. When I directly pass the particular user id, I get the data. But when I pass it using variable it shows null.
My code is as follows:
import 'package:cloud_firestore/cloud_firestore.dart'; import 'package:flutter/material.dart'; import 'package:firebase_auth/firebase_auth.dart'; import '../services/crud.dart'; class test extends StatefulWidget { @override _testState createState() => _testState(); } class _testState extends State<test> { String userID=""; @override void initState() { super.initState(); ///get current user and assign his id FirebaseAuth.instance.currentUser().then((FirebaseUser user) { setState(() { userID = user.uid; print(userID); }); }); } @override Widget build(BuildContext context) { return SingleChildScrollView( child: Column( children: <Widget>[ StreamBuilder( stream: Firestore.instance.collection('users').document(userID).snapshots(), builder: (context,snapshot){ if (!snapshot.hasData) return const Text("Loading..."); else return Container( child: Column( children: <Widget>[ Text(snapshot.data["name"]), Text(snapshot.data["email"]), Text(snapshot.data["phone"].toString()), ], ), ); }, ) ], ) ); } }
When I'm using the following line of code with specifying uid it shows the result :
stream: Firestore.instance.collection('users').document('d6DshRomJMkIe9mAARAi').snapshots(),
But it does not work when I pass userID inside the document(). Even though userID contains the actual id of the logged-in user.
stream: Firestore.instance.collection('users').document(userID).snapshots(),
The error says :
NoSuchMethodError: The method '[]' was called on null. Receiver: null Tried calling []("name")