How to get or pick local GIF file from device
566
you can use file_picker package and set the allowedExtensions
to gif
.
allowedExtensions: ['gif']
here is an example:
import 'dart:io';
import 'package:flutter/material.dart';
import 'package:file_picker/file_picker.dart';
void main() {
runApp(MaterialApp(
home: MyApp(),
));
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
var _selectedFile;
String customAppLogoName;
_openFileManager() async {
FilePickerResult result = await FilePicker.platform.pickFiles(
type: FileType.custom,
allowedExtensions: ['gif'],
);
if (result != null) {
PlatformFile selectedFile = result.files.first;
setState(() {
_selectedFile = File(selectedFile.path);
});
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.white,
appBar: AppBar(
title: Text("File Picker"),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
_openFileManager();
},
child: Icon(Icons.image),
),
body: Center(
child: Container(
child: _selectedFile == null
? Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Icon(
Icons.broken_image,
size: 100,
),
Text('no image'),
],
)
: Image(
image: FileImage(_selectedFile),
),
),
),
);
}
}
result:
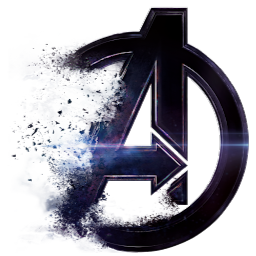
Author by
Alpit Panchal
Updated on November 26, 2022Comments
-
Alpit Panchal over 1 year
I have done lots of R&D about the pick-only GIF file and all give results only display or showing GIF files but I want to only pick GIF files from a local device like images or videos.
-
Alpit Panchal almost 3 yearsThanks for the reply, It's working but also the image file selectable.