How to get screen height in flutter
Solution 1
Hy tony i found answer for your question you need to add safeArea for it
class BaseLayout extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: SafeArea(
child: Scaffold(
body: Container(
height: MediaQuery.of(context).size.height,
color: Colors.red,
),
),
),
);
}
}
Solution 2
Edited
You have to povide it above the context of MediaQuery's context:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: BaseLayout()
);
}
}
class BaseLayout extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Container(
height: MediaQuery.of(context).size.height,
color: Colors.red,
),
),
);
}
}
create an own class and your error should be gone.
original answer
Do you have MaterialApp in your widget tree? MaterialApp provides MediaQuery.
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
body:WhateverWidget
);
}
}
By the way... the solution is in your error code ;)
This can happen because you have not added a WidgetsApp, CupertinoApp, or MaterialApp widget (those widgets introduce a MediaQuery), or it can happen if the context you use comes from a widget above those widgets.)
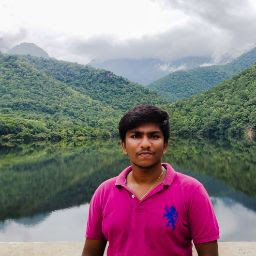
Comments
-
Tony wilson jesuraj over 1 year
Hi Im just trying to get height using
MediaQuery.of(context).size.height
but gettingFlutterError (No MediaQuery widget ancestor found. MyApp widgets require a MediaQuery widget ancestor. The specific widget that could not find a MediaQuery ancestor was: MyApp The ownership chain for the affected widget is: "MyApp ← [root]" No MediaQuery ancestor could be found starting from the context that was passed to MediaQuery.of(). This can happen because you have not added a WidgetsApp, CupertinoApp, or MaterialApp widget (those widgets introduce a MediaQuery), or it can happen if the context you use comes from a widget above those widgets.)
class BaseLayout extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( body: Container( height: MediaQuery.of(context).size.height, color: Colors.red, ), ), ); } }
-
Anmol Mishra over 2 years
-
-
Tony wilson jesuraj over 2 yearsHi @MCB I have MaterialApp widget in my code still its showing me an error. updated my code you can refer with it