How to get the last part of a string in JavaScript?
50,054
Solution 1
One way:
var lastPart = url.split("/").pop();
Solution 2
Assuming there is no trailing slash, you could get it like this:
var url = "http://www.mysite.com/category/action";
var parts = url.split("/");
alert(parts[parts.length-1]);
However, if there can be a trailing slash, you could use the following:
var url = "http://www.mysite.com/category/action/";
var parts = url.split("/");
if (parts[parts.length-1].length==0){
alert(parts[parts.length-2]);
}else{
alert(parts[parts.length-1]);
}
Solution 3
str.substring(str.lastIndexOf("/") + 1)
Though if your URL could contain a query or fragment, you might want to do
var end = str.lastIndexOf("#");
if (end >= 0) { str = str.substring(0, end); }
end = str.lastIndexOf("?");
if (end >= 0) { str = str.substring(0, end); }
first to make sure you have a URL with the path at the end.
Solution 4
Or the regex way:
var lastPart = url.replace(/.*\//, ""); //tested in FF 3
OR
var lastPart = url.match(/[^/]*$/)[0]; //tested in FF 3
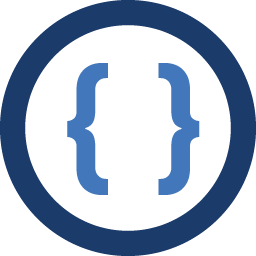
Author by
Admin
Updated on May 29, 2020Comments
-
Admin almost 4 years
My url will look like this:
http://www.example.com/category/action
How can I get the word "action". This last part of the url (after the last forward slash "/") will be different each time. So whether its "action" or "adventure", etc. how can I always get the word after the last closing forward slash?
-
Ates Goral almost 13 yearsMore reliable resource: developer.mozilla.org/en/JavaScript/Reference/Global_Objects/…
-
kimbaudi almost 5 yearsin case the url has a trailing slash (i.e.,
http://www.example.com/category/action/
), we can apply a filter empty parts:var lastPart = url.split('/').filter(e => e).pop()
. Also, I prefer to useslice(-1)
instead ofpop()
to return the last part becausepop()
returnsundefined
whileslice(-1)
will return empty array. So I would do:var lastPart = url.split('/').filter(e => e).slice(-1)
-
kimbaudi almost 5 yearsto handle trailing slash, i would filter out empty parts after splitting by
/
. Then you can get the last part usingslice(-1)
. So it would be:var lastPart = url.split('/').filter(e => e).slice(-1);