How to get video duration, dimension and size in PHP?
Solution 1
getID3 supports video formats. See: http://getid3.sourceforge.net/
Edit: So, in code format, that'd be like:
include_once('pathto/getid3.php');
$getID3 = new getID3;
$file = $getID3->analyze($filename);
echo("Duration: ".$file['playtime_string'].
" / Dimensions: ".$file['video']['resolution_x']." wide by ".$file['video']['resolution_y']." tall".
" / Filesize: ".$file['filesize']." bytes<br />");
Note: You must include the getID3 classes before this will work! See the above link.
Edit: If you have the ability to modify the PHP installation on your server, a PHP extension for this purpose is ffmpeg-php. See: http://ffmpeg-php.sourceforge.net/
Solution 2
If you have FFMPEG installed on your server (http://www.mysql-apache-php.com/ffmpeg-install.htm), it is possible to get the attributes of your video using the command "-vstats" and parsing the result with some regex - as shown in the example below. Then, you need the PHP function filesize() to get the size.
$ffmpeg_path = 'ffmpeg'; //or: /usr/bin/ffmpeg , or /usr/local/bin/ffmpeg - depends on your installation (type which ffmpeg into a console to find the install path)
$vid = 'PATH/TO/VIDEO'; //Replace here!
if (file_exists($vid)) {
$finfo = finfo_open(FILEINFO_MIME_TYPE);
$mime_type = finfo_file($finfo, $vid); // check mime type
finfo_close($finfo);
if (preg_match('/video\/*/', $mime_type)) {
$video_attributes = _get_video_attributes($vid, $ffmpeg_path);
print_r('Codec: ' . $video_attributes['codec'] . '<br/>');
print_r('Dimension: ' . $video_attributes['width'] . ' x ' . $video_attributes['height'] . ' <br/>');
print_r('Duration: ' . $video_attributes['hours'] . ':' . $video_attributes['mins'] . ':'
. $video_attributes['secs'] . '.' . $video_attributes['ms'] . '<br/>');
print_r('Size: ' . _human_filesize(filesize($vid)));
} else {
print_r('File is not a video.');
}
} else {
print_r('File does not exist.');
}
function _get_video_attributes($video, $ffmpeg) {
$command = $ffmpeg . ' -i ' . $video . ' -vstats 2>&1';
$output = shell_exec($command);
$regex_sizes = "/Video: ([^,]*), ([^,]*), ([0-9]{1,4})x([0-9]{1,4})/"; // or : $regex_sizes = "/Video: ([^\r\n]*), ([^,]*), ([0-9]{1,4})x([0-9]{1,4})/"; (code from @1owk3y)
if (preg_match($regex_sizes, $output, $regs)) {
$codec = $regs [1] ? $regs [1] : null;
$width = $regs [3] ? $regs [3] : null;
$height = $regs [4] ? $regs [4] : null;
}
$regex_duration = "/Duration: ([0-9]{1,2}):([0-9]{1,2}):([0-9]{1,2}).([0-9]{1,2})/";
if (preg_match($regex_duration, $output, $regs)) {
$hours = $regs [1] ? $regs [1] : null;
$mins = $regs [2] ? $regs [2] : null;
$secs = $regs [3] ? $regs [3] : null;
$ms = $regs [4] ? $regs [4] : null;
}
return array('codec' => $codec,
'width' => $width,
'height' => $height,
'hours' => $hours,
'mins' => $mins,
'secs' => $secs,
'ms' => $ms
);
}
function _human_filesize($bytes, $decimals = 2) {
$sz = 'BKMGTP';
$factor = floor((strlen($bytes) - 1) / 3);
return sprintf("%.{$decimals}f", $bytes / pow(1024, $factor)) . @$sz[$factor];
}
Solution 3
If you use Wordpress you can just use the wordpress build in function with the video id provided wp_get_attachment_metadata($videoID):
wp_get_attachment_metadata($videoID);
helped me a lot. thats why i'm posting it, although its just for wordpress users.
Solution 4
https://github.com/JamesHeinrich/getID3 download getid3 zip and than only getid3 named folder copy paste in project folder and use it as below show...
<?php
require_once('/fire/scripts/lib/getid3/getid3/getid3.php');
$getID3 = new getID3();
$filename="/fire/My Documents/video/ferrari1.mpg";
$fileinfo = $getID3->analyze($filename);
$width=$fileinfo['video']['resolution_x'];
$height=$fileinfo['video']['resolution_y'];
echo $fileinfo['video']['resolution_x']. 'x'. $fileinfo['video']['resolution_y'];
echo '<pre>';print_r($fileinfo);echo '</pre>';
?>
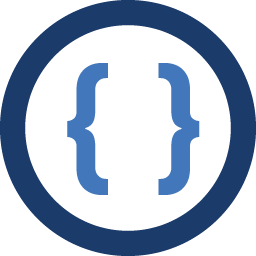
Admin
Updated on July 02, 2020Comments
-
Admin almost 4 years
I want to know how to get the duration, dimension and size of uploaded video file in PHP. The file can be in any video format.
-
Admin over 13 yearsHi aendrew,Thanks but this workes well for the audio file but not working correctly on the video files like mp4,wmv etc... Please let me know any other solution apart from this.
-
aendra over 13 yearsUse a different library, then, or a PHP extension like ffmpeg-php (ffmpeg-php.sourceforge.net)
-
gkd almost 10 yearswhat is the problem with getID3 and ffmpeg ?? why they are not making UI proper so that reading that become very user friendly... got fade up by looking at home page of getID3 only...
-
aendra almost 10 years@GhanshyamDobariya Different tools are useful for different uses. getID3 would be significantly less useful to me if it had a UI.
-
kmoney12 almost 9 yearsI tested this with an FLV file that had 480 res and the
$file['video']['resolution_x']
gave meint(16)
. I was able to get the correct video width using$width=$file['flv']['meta']['onMetaData']['width'];
-
John Erck almost 9 yearsIf you're using composer:
{"require":{"james-heinrich/getid3": ">=1.9.9"}}
then runphp composer.phar update
. -
1owk3y about 7 yearsThanks so much for this! I found that the provided regex fell short because my test videos had an extra comma in the codec section. That said, here's a fix for anyone with the same problem:
$regex_sizes = "/Video: ([^\r\n]*), ([^,]*), ([0-9]{1,4})x([0-9]{1,4})/";
also, my ffmpeg path was:/usr/local/bin/ffmpeg
(typewhich ffmpeg
into a console to find the install path). Here's a Regexr sample: regexr.com/3fs8d -
FosAvance almost 7 yearsThis one helped me a lot :D
-
Fokrule over 5 yearsBest one. Thanks a lot
-
Manish gour about 3 yearsWorked well with MOV, FLV and MP4, thanks.
-
WilliamK over 2 yearsWhen checking a file on Amazon S3 I get no result. Does this only work locally?