how to hide column in google charts table
Solution 1
Instead of drawing the DataTable, you have to create an in-between DataView object where you filter the original data. Something like this:
//dataResponse is your Datatable with A,B,C,D columns
var view = new google.visualization.DataView(dataResponse);
view.setColumns([0,1,3]); //here you set the columns you want to display
//Visualization Go draw!
visualizationPlot.draw(view, options);
The hideColumns method is maybe better instead of setColumns, choose yourself!
Cheers
Solution 2
Here's an alternative using a ChartWrapper instead of a chart.
var opts = {
"containerId": "chart_div",
"dataTable": datatable,
"chartType": "Table",
"options": {"title": "Now you see the columns, now you don't!"}
}
var chartwrapper = new google.visualization.ChartWrapper(opts);
// set the columns to show
chartwrapper.setView({'columns': [0, 1, 4, 5]});
chartwrapper.draw();
If you use a ChartWrapper, you can easily add a function to change the hidden columns, or show all the columns. To show all the columns, pass null
as the value of 'columns'
. For instance, using jQuery,
$('button').click(function() {
// use your preferred method to get an array of column indexes or null
var columns = eval($(this).val());
chartwrapper.setView({'columns': columns});
chartwrapper.draw();
});
In your html,
<button value="[0, 1, 3]" >Hide columns</button>
<button value="null">Expand All</button>
(Note: eval
used for conciseness. Use what suits your code. It's beside the point.)
Solution 3
var view = new google.visualization.DataView(dataTable); //datatable contains col and rows
view.setColumns([0,1,3,4]); //only show these column
chart.draw(view, options); //pass the view to draw chat
Solution 4
You can do this with CSS.
"#table_div" is the div my table is wrapped in. I use this because there a multiple tables on the same page.
#table_div .google-visualization-table table.google-visualization-table-table
td:nth-child(1),th:nth-child(1){
display:none;
}
I also have an event handler on the chart's rows, and can still pick up the data from the hidden column.
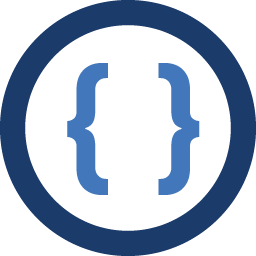
Admin
Updated on July 26, 2020Comments
-
Admin almost 4 years
I've got a Google Charts Table that displays a couple of columns and rows. Say for instance we have 4 columns(A,B,C,D respectively). How would I be able to still load column C's values, but just hide the column so that it's not getting displayed?
Thanks in advance!
-
Myx almost 12 yearsis there a way to have this behavior happen when someone clicks on a link? For example, by default, I want to hide some columns (using view.hideColumns). Then I want to have an 'Expand' link above the table that when the user clicks on it, the remaining columns are exposed (using view.setColumns). I'm also open to suggestions for better implementations of this behavior.